mirror of
https://github.com/dart-lang/sdk
synced 2024-09-04 16:03:44 +00:00
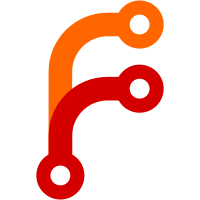
* Merge ClassTable and SharedClassTable back together; * Simplify handling of multiple arrays growing in sync; * Refactor how reload deals with ClassTable. The last change is the most important because it makes it much easier to reason about the code. We move away from copying bits and pieces of the class table and shared class table into reload contexts. Having two class table fields in the isolate group makes it easier to reason about. One field contains program class table (one modified by kernel loader and accessed by various program structure cid lookups) and heap walk class table (used by GC visitors). Normally these two fields point to the same class table, but during hot reload we temporary split them apart: original class table is kept as a heap walk class table, while program class table is replaced by a clone and updated by reload. If reload succeeds we drop original class table and set program class table as heap walk one. If reload fails we drop the program class table and restore original one from heap walk table. TEST=ci Cq-Include-Trybots: luci.dart.try:vm-kernel-reload-linux-release-x64-try,vm-kernel-reload-linux-debug-x64-try,vm-kernel-reload-rollback-linux-debug-x64-try,vm-kernel-reload-rollback-linux-release-x64-try,vm-kernel-linux-debug-x64-try,vm-kernel-precomp-tsan-linux-release-x64-try,vm-kernel-tsan-linux-release-x64-try,vm-kernel-precomp-asan-linux-release-x64-try,vm-kernel-asan-linux-release-x64-try Change-Id: I8b66259fcc474dea7dd2af063e4772df99be06c4 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/258361 Commit-Queue: Slava Egorov <vegorov@google.com> Reviewed-by: Ryan Macnak <rmacnak@google.com>
64 lines
1.7 KiB
C++
64 lines
1.7 KiB
C++
// Copyright (c) 2017, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_PLATFORM_ALLOCATION_H_
|
|
#define RUNTIME_PLATFORM_ALLOCATION_H_
|
|
|
|
#include "platform/address_sanitizer.h"
|
|
#include "platform/assert.h"
|
|
|
|
namespace dart {
|
|
|
|
void* calloc(size_t n, size_t size);
|
|
void* malloc(size_t size);
|
|
void* realloc(void* ptr, size_t size);
|
|
|
|
// Stack allocated objects subclass from this base class. Objects of this type
|
|
// cannot be allocated on either the C or object heaps. Destructors for objects
|
|
// of this type will not be run unless the stack is unwound through normal
|
|
// program control flow.
|
|
class ValueObject {
|
|
public:
|
|
ValueObject() {}
|
|
~ValueObject() {}
|
|
|
|
private:
|
|
DISALLOW_ALLOCATION();
|
|
DISALLOW_COPY_AND_ASSIGN(ValueObject);
|
|
};
|
|
|
|
// Static allocated classes only contain static members and can never
|
|
// be instantiated in the heap or on the stack.
|
|
class AllStatic {
|
|
private:
|
|
DISALLOW_ALLOCATION();
|
|
DISALLOW_IMPLICIT_CONSTRUCTORS(AllStatic);
|
|
};
|
|
|
|
class MallocAllocated {
|
|
public:
|
|
MallocAllocated() {}
|
|
|
|
// Intercept operator new to produce clearer error messages when we run out
|
|
// of memory. Don't do this when running under ASAN so it can continue to
|
|
// check malloc/new/new[] are paired with free/delete/delete[] respectively.
|
|
#if !defined(USING_ADDRESS_SANITIZER)
|
|
void* operator new(size_t size) {
|
|
return dart::malloc(size);
|
|
}
|
|
|
|
void* operator new[](size_t size) {
|
|
return dart::malloc(size);
|
|
}
|
|
|
|
void operator delete(void* pointer) { ::free(pointer); }
|
|
|
|
void operator delete[](void* pointer) { ::free(pointer); }
|
|
#endif
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_PLATFORM_ALLOCATION_H_
|