mirror of
https://github.com/dart-lang/sdk
synced 2024-09-19 23:51:47 +00:00
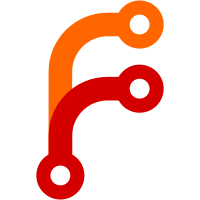
CallSpecializer::TryInlineInstanceSetter was using IsReceiver to determine type check could be skipped. However it is incorrect to use IsReceiver, because it uses SSA notion of the receiver - rather than a syntactic notion and all strong mode guarantees apply at syntactic level. Additionally this CL expands support for exactness tracking to PropertySet operations and uses it to eliminate checks on setters. Change-Id: Idce559c4ca4c88f5788d3b6b9955b5af076bd7eb Reviewed-on: https://dart-review.googlesource.com/72442 Commit-Queue: Vyacheslav Egorov <vegorov@google.com> Reviewed-by: Samir Jindel <sjindel@google.com>
57 lines
1.3 KiB
Dart
57 lines
1.3 KiB
Dart
// Copyright (c) 2018, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
//
|
|
// VMOptions=--no-background-compilation --enable-inlining-annotations --optimization-counter-threshold=10
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
const NeverInline = "NeverInline";
|
|
|
|
class A<T> {
|
|
T field;
|
|
|
|
@NeverInline
|
|
set property(T v) {}
|
|
|
|
@NeverInline
|
|
void method(T x) {}
|
|
|
|
@NeverInline
|
|
void testMethod(bool violateType) {
|
|
A<dynamic> x = this;
|
|
x.method(violateType ? 10 : "10");
|
|
}
|
|
|
|
@NeverInline
|
|
void testSetter(bool violateType) {
|
|
A<dynamic> x = this;
|
|
x.property = violateType ? 10 : "10";
|
|
}
|
|
|
|
@NeverInline
|
|
void testField(bool violateType) {
|
|
A<dynamic> x = this;
|
|
x.field = violateType ? 10 : "10";
|
|
}
|
|
}
|
|
|
|
@NeverInline
|
|
void loop(A<String> obj, bool violateType) {
|
|
for (var i = 0; i < 100; i++) {
|
|
obj.testMethod(violateType);
|
|
obj.testSetter(violateType);
|
|
obj.testField(violateType);
|
|
}
|
|
}
|
|
|
|
void main() {
|
|
A<num>().field = 10;
|
|
final obj = A<String>();
|
|
loop(obj, false);
|
|
loop(obj, false);
|
|
Expect.throwsTypeError(() => obj.testMethod(true));
|
|
Expect.throwsTypeError(() => obj.testSetter(true));
|
|
Expect.throwsTypeError(() => obj.testField(true));
|
|
}
|