mirror of
https://github.com/dart-lang/sdk
synced 2024-09-19 20:41:45 +00:00
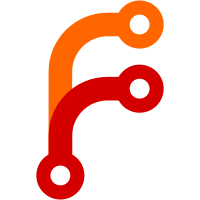
TableTree improvements: - Row's have flex-box divs in each table cell. - To represent depth in tree a colored vertical bar for each depth level is shown - Expanding tree nodes is now asynchronous and animates. - Change cursor during tree expansion. Observatory Profiler improvements: - Improved appearance. - Support for inclusive (main first) call trees. - Replace tooltip hover with info toggle. - Visual icon attributes for code, functions and call tree nodes. - Fetching / Load status display. - Cleaned up Code and Function kinds. Profiler improvements: - When not profiling the VM (--profile-vm) only walk the stack when we are in Dart code or have exited Dart code. This removes some native stack walker noise. - Build inclusive (main first) call trees and send across service. Notes for analyzer team: Analyzer command line: ./out/ReleaseIA32/dart --keep-code --trace-profiler --profile-depth=128 --observe out/ReleaseIA32/dart-sdk/bin/snapshots/dartanalyzer.dart.snapshot --dart-sdk=out/ReleaseIA32/dart-sdk pkg/compiler/lib/src/dart2js.dart Profiler settings: - Tags: None or VM - Mode: Function - Direction: Bottom up R=asiva@google.com, rmacnak@google.com Review URL: https://codereview.chromium.org//965593002 git-svn-id: https://dart.googlecode.com/svn/branches/bleeding_edge/dart@44152 260f80e4-7a28-3924-810f-c04153c831b5
49 lines
1.1 KiB
C++
49 lines
1.1 KiB
C++
// Copyright (c) 2015, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef VM_SCOPE_TIMER_H_
|
|
#define VM_SCOPE_TIMER_H_
|
|
|
|
#include "platform/globals.h"
|
|
|
|
namespace dart {
|
|
|
|
// Simple utility class for timing a block of code.
|
|
class ScopeTimer : public ValueObject {
|
|
public:
|
|
explicit ScopeTimer(const char* name, bool enabled = true)
|
|
: enabled_(enabled),
|
|
name_(name),
|
|
start_(0) {
|
|
if (!enabled_) {
|
|
return;
|
|
}
|
|
start_ = OS::GetCurrentTimeMicros();
|
|
}
|
|
|
|
int64_t GetElapsed() const {
|
|
int64_t end = OS::GetCurrentTimeMicros();
|
|
ASSERT(end >= start_);
|
|
return end - start_;
|
|
}
|
|
|
|
~ScopeTimer() {
|
|
if (!enabled_) {
|
|
return;
|
|
}
|
|
int64_t elapsed = GetElapsed();
|
|
double seconds = MicrosecondsToSeconds(elapsed);
|
|
OS::Print("%s: %f seconds (%" Pd64 " \u00B5s)\n", name_, seconds, elapsed);
|
|
}
|
|
|
|
private:
|
|
const bool enabled_;
|
|
const char* name_;
|
|
int64_t start_;
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // VM_SCOPE_TIMER_H_
|