mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 08:24:39 +00:00
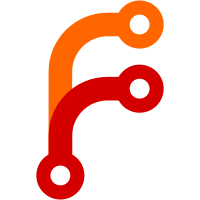
This CL adds FFI leaf calls by adding `lookupFunction(.., isLeaf)` and `_asFunctionInternal(.., isLeaf)`, which generate FFI leaf calls. These calls skip a lot of the usual frame building and generated <-> native transition overhead. `benchmark/FfiCall/` shows a 1.1x - 4.3x speed-up between the regular FFI calls and their leaf call counterparts (JIT, x64, release). TEST=Adds `tests/ffi{,_2}/vmspecific_leaf_call_test.dart`. Tested FFI tests. Closes: https://github.com/dart-lang/sdk/issues/36707 Cq-Include-Trybots: luci.dart.try:vm-precomp-ffi-qemu-linux-release-arm-try,vm-ffi-android-release-arm64-try,vm-ffi-android-release-arm-try,vm-ffi-android-product-arm64-try,vm-ffi-android-product-arm-try,vm-ffi-android-debug-arm64-try,vm-ffi-android-debug-arm-try,vm-kernel-linux-debug-ia32-try,vm-kernel-win-debug-ia32-try,vm-kernel-win-debug-x64-try,vm-kernel-win-release-x64-try,vm-kernel-mac-debug-x64-try,vm-kernel-precomp-nnbd-mac-release-simarm64-try,vm-kernel-precomp-android-release-arm64-try,vm-kernel-precomp-asan-linux-release-x64-try,vm-kernel-precomp-linux-release-simarm_x64-try,vm-kernel-precomp-obfuscate-linux-release-x64-try,vm-kernel-precomp-ubsan-linux-release-x64-try,vm-kernel-precomp-tsan-linux-release-x64-try,vm-kernel-precomp-win-release-x64-try,vm-precomp-ffi-qemu-linux-release-arm-try,vm-kernel-reload-rollback-linux-debug-x64-try,vm-kernel-reload-linux-debug-x64-try Bug: https://github.com/dart-lang/sdk/issues/36707 Change-Id: Id8824f36b0006bf09951207bd004356fe6e9f46e Cq-Do-Not-Cancel-Tryjobs: true Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/179768 Commit-Queue: Clement Skau <cskau@google.com> Reviewed-by: Daco Harkes <dacoharkes@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com>
47 lines
1.3 KiB
Dart
47 lines
1.3 KiB
Dart
// Copyright (c) 2019, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// @dart = 2.9
|
|
|
|
import 'dart:ffi';
|
|
|
|
import 'dylib_utils.dart';
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
final ffiTestFunctions = dlopenPlatformSpecific("ffi_test_functions");
|
|
|
|
typedef NativeCallbackTest = Int32 Function(Pointer);
|
|
typedef NativeCallbackTestFn = int Function(Pointer);
|
|
|
|
class CallbackTest {
|
|
final String name;
|
|
final Pointer callback;
|
|
final void Function() afterCallbackChecks;
|
|
final bool isLeaf;
|
|
|
|
CallbackTest(this.name, this.callback, {this.isLeaf: false})
|
|
: afterCallbackChecks = noChecks {}
|
|
CallbackTest.withCheck(this.name, this.callback, this.afterCallbackChecks,
|
|
{this.isLeaf: false}) {}
|
|
|
|
void run() {
|
|
final NativeCallbackTestFn tester = isLeaf
|
|
? ffiTestFunctions.lookupFunction<NativeCallbackTest,
|
|
NativeCallbackTestFn>("Test$name", isLeaf: true)
|
|
: ffiTestFunctions.lookupFunction<NativeCallbackTest,
|
|
NativeCallbackTestFn>("Test$name", isLeaf: false);
|
|
|
|
final int testCode = tester(callback);
|
|
|
|
if (testCode != 0) {
|
|
Expect.fail("Test $name failed.");
|
|
}
|
|
|
|
afterCallbackChecks();
|
|
}
|
|
}
|
|
|
|
void noChecks() {}
|