mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 16:41:07 +00:00
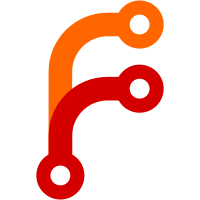
Closes: https://github.com/dart-lang/sdk/issues/38158 This CL implements unaligned access for float/double on arm32, but does not expose it in an API. Rather it only uses these loads/stores inside the getters and setters of packed structs. Bug: https://github.com/dart-lang/sdk/issues/45009 Besides unaligned access for float/double, this CL is mostly a CFE change. The only VM change is reading the packing in the `_FfiStructLayout` annotation. The implementation of using the packing in the VM, and analyzer changes have landed separately in earlier CLs. tools/test.py ffi ffi_2 TEST=tests/ffi(_2)/(.*)by_value_(*.)_test.dart Change-Id: Ic3106ecc626d2e30674a49bf03f65ae12d2b3502 Cq-Include-Trybots: luci.dart.try:dart-sdk-linux-try,dart-sdk-mac-try,dart-sdk-win-try,vm-ffi-android-debug-arm-try,vm-ffi-android-debug-arm64-try,vm-kernel-asan-linux-release-x64-try,vm-kernel-mac-debug-x64-try,vm-kernel-linux-debug-ia32-try,vm-kernel-linux-debug-x64-try,vm-kernel-nnbd-linux-debug-x64-try,vm-kernel-nnbd-linux-debug-ia32-try,vm-kernel-nnbd-mac-release-x64-try,vm-kernel-nnbd-win-debug-x64-try,vm-kernel-precomp-linux-debug-x64-try,vm-kernel-precomp-linux-debug-simarm_x64-try,vm-kernel-precomp-nnbd-linux-debug-x64-try,vm-kernel-precomp-win-release-x64-try,vm-kernel-reload-linux-debug-x64-try,vm-kernel-reload-rollback-linux-debug-x64-try,vm-kernel-win-debug-x64-try,vm-kernel-win-debug-ia32-try,vm-precomp-ffi-qemu-linux-release-arm-try,vm-kernel-precomp-obfuscate-linux-release-x64-try,vm-kernel-msan-linux-release-x64-try,vm-kernel-precomp-msan-linux-release-x64-try,vm-kernel-precomp-android-release-arm_x64-try,analyzer-analysis-server-linux-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/186143 Reviewed-by: Aske Simon Christensen <askesc@google.com>
41 lines
1.1 KiB
Dart
41 lines
1.1 KiB
Dart
// Copyright (c) 2021, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
//
|
|
// SharedObjects=ffi_test_functions
|
|
|
|
import 'dart:ffi';
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
import 'dylib_utils.dart';
|
|
|
|
// Reuse struct definitions.
|
|
import 'function_structs_by_value_generated_test.dart';
|
|
|
|
void main() {
|
|
testSizeOfC();
|
|
testSizeOfDart();
|
|
}
|
|
|
|
final ffiTestFunctions = dlopenPlatformSpecific("ffi_test_functions");
|
|
|
|
final sizeOfStruct3BytesPackedInt =
|
|
ffiTestFunctions.lookupFunction<Uint64 Function(), int Function()>(
|
|
"SizeOfStruct3BytesPackedInt");
|
|
|
|
void testSizeOfC() {
|
|
Expect.equals(3, sizeOfStruct3BytesPackedInt());
|
|
}
|
|
|
|
void testSizeOfDart() {
|
|
// No packing needed to get to 3 bytes.
|
|
Expect.equals(3, sizeOf<Struct3BytesHomogeneousUint8>());
|
|
|
|
// Contents 3 bytes, but alignment forces it to be 4 bytes.
|
|
Expect.equals(4, sizeOf<Struct3BytesInt2ByteAligned>());
|
|
|
|
// Alignment gets the same content back to 3 bytes.
|
|
Expect.equals(3, sizeOf<Struct3BytesPackedInt>());
|
|
}
|