mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
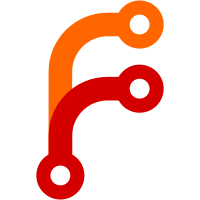
The API for pkg/front_end is still in flux so we want to avoid having any packages depend directly on it (other than analyzer and back-ends). This CL re-exports some of the critical pieces of front_end needed by analyzer clients so that those clients can access them via analyzer, without having to directly depend on front_end. It also updates pkg/analyzer_plugin to make use of those re-exports. R=scheglov@google.com Review-Url: https://codereview.chromium.org/2993123002 .
56 lines
2 KiB
Dart
56 lines
2 KiB
Dart
// Copyright (c) 2017, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'package:analyzer/src/codegen/tools.dart';
|
|
import 'package:path/path.dart' as path;
|
|
|
|
import 'api.dart';
|
|
import 'codegen_dart_protocol.dart';
|
|
import 'from_html.dart';
|
|
import 'implied_types.dart';
|
|
|
|
GeneratedFile target(bool responseRequiresRequestTime) =>
|
|
new GeneratedFile('lib/protocol/protocol_common.dart', (String pkgPath) {
|
|
CodegenCommonVisitor visitor = new CodegenCommonVisitor(
|
|
path.basename(pkgPath),
|
|
responseRequiresRequestTime,
|
|
readApi(pkgPath));
|
|
return visitor.collectCode(visitor.visitApi);
|
|
});
|
|
|
|
/**
|
|
* A visitor that produces Dart code defining the common types associated with
|
|
* the API.
|
|
*/
|
|
class CodegenCommonVisitor extends CodegenProtocolVisitor {
|
|
/**
|
|
* Initialize a newly created visitor to generate code in the package with the
|
|
* given [packageName] corresponding to the types in the given [api] that are
|
|
* common to multiple protocols.
|
|
*/
|
|
CodegenCommonVisitor(
|
|
String packageName, bool responseRequiresRequestTime, Api api)
|
|
: super(packageName, responseRequiresRequestTime, api);
|
|
|
|
@override
|
|
void emitImports() {
|
|
writeln("import 'dart:convert' hide JsonDecoder;");
|
|
writeln();
|
|
writeln("import 'package:analyzer/src/generated/utilities_general.dart';");
|
|
writeln("import 'package:$packageName/protocol/protocol.dart';");
|
|
writeln(
|
|
"import 'package:$packageName/src/protocol/protocol_internal.dart';");
|
|
}
|
|
|
|
@override
|
|
List<ImpliedType> getClassesToEmit() {
|
|
List<ImpliedType> types = impliedTypes.values.where((ImpliedType type) {
|
|
ApiNode node = type.apiNode;
|
|
return node is TypeDefinition && node.isExternal;
|
|
}).toList();
|
|
types.sort((first, second) =>
|
|
capitalize(first.camelName).compareTo(capitalize(second.camelName)));
|
|
return types;
|
|
}
|
|
}
|