mirror of
https://github.com/dart-lang/sdk
synced 2024-09-15 22:31:50 +00:00
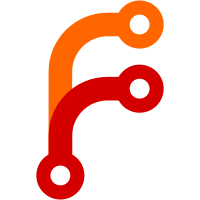
This linked in kernel service dill file will be used to load the kernel isolate if the attempt to lookup the kernel service snapshot fails. The kernel service snapshot is looked up in the following order. 1. If the "--dfe" option is specified, the file specified is used. 2. If the kernel service snapshot is found next to the executable, then it is used. 3. If the kernel service snapshot is found in the "snapshots" directory next to the executable, then it is used. Change-Id: I5a0e757eb27b26a274b22b4bc36350fee59a100f Reviewed-on: https://dart-review.googlesource.com/32446 Reviewed-by: Ryan Macnak <rmacnak@google.com> Reviewed-by: Siva Annamalai <asiva@google.com> Commit-Queue: Siva Chandra <sivachandra@google.com>
78 lines
2.1 KiB
Python
Executable file
78 lines
2.1 KiB
Python
Executable file
#!/usr/bin/env python
|
|
#
|
|
# Copyright (c) 2018, the Dart project authors. Please see the AUTHORS file
|
|
# for details. All rights reserved. Use of this source code is governed by a
|
|
# BSD-style license that can be found in the LICENSE file.
|
|
|
|
# Script to create a cc file with uint8 array of bytes corresponding to the
|
|
# content of given a dill file.
|
|
|
|
import optparse
|
|
import sys
|
|
|
|
# Option => Help mapping.
|
|
OPTION_MAP = {
|
|
'dill_file': 'Path to the input dill file.',
|
|
'data_symbol': 'The C name for the data array.',
|
|
'size_symbol': 'The C name for the data size variable.',
|
|
'output': 'Path to the generated cc file.',
|
|
}
|
|
|
|
|
|
def BuildOptionParser():
|
|
parser = optparse.OptionParser()
|
|
for opt, help_text in OPTION_MAP.iteritems():
|
|
parser.add_option('--%s' % opt, type='string', help=help_text)
|
|
return parser
|
|
|
|
|
|
def ValidateOptions(options):
|
|
for opt in OPTION_MAP.keys():
|
|
if getattr(options, opt) is None:
|
|
sys.stderr.write('--%s option not specified.\n' % opt)
|
|
return False
|
|
return True
|
|
|
|
|
|
def WriteData(input_filename, data_symbol, output_file):
|
|
output_file.write('uint8_t %s[] = {\n' % data_symbol)
|
|
with open(input_filename, 'rb') as f:
|
|
first = True
|
|
size = 0
|
|
for byte in f.read():
|
|
if first:
|
|
output_file.write(' %d' % ord(byte))
|
|
first = False
|
|
else:
|
|
output_file.write(',\n %d' % ord(byte))
|
|
size += 1
|
|
output_file.write('\n};\n')
|
|
return size
|
|
|
|
|
|
def WriteSize(size_symbol, size, output_file):
|
|
output_file.write('intptr_t %s = %d;\n' % (size_symbol, size))
|
|
|
|
|
|
def Main():
|
|
opt_parser = BuildOptionParser()
|
|
(options, args) = opt_parser.parse_args()
|
|
if not ValidateOptions(options):
|
|
opt_parser.print_help()
|
|
return 1
|
|
if args:
|
|
sys.stderr.write('Unknown args: "%s"\n' % str(args))
|
|
parser.print_help()
|
|
return 1
|
|
|
|
with open(options.output, 'w') as output_file:
|
|
output_file.write('#include <stdint.h>\n')
|
|
output_file.write('extern "C" {\n')
|
|
size = WriteData(options.dill_file, options.data_symbol, output_file)
|
|
WriteSize(options.size_symbol, size, output_file)
|
|
output_file.write("}")
|
|
|
|
|
|
if __name__ == '__main__':
|
|
sys.exit(Main())
|