mirror of
https://github.com/dart-lang/sdk
synced 2024-09-15 22:19:49 +00:00
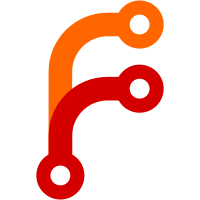
Before this CL, `FfiNative`s were first transformed to `asFunction` calls, which were then immediately transformed to `_asFunctionInternal` calls. This caused the the static checks to be done in two steps, the second step happening after the first transform. It is cleaner to first do all checks. This refactoring enables implementing `_asFunctionInternal` variants for `FfiNative`s that don't use a `Pointer` for the address. Besides the transform change, this CL - moves shared logic over to pkg/vm/lib/transformations/ffi/common.dart, - splits up the ffi-native tests in to positive and negative tests, and - adds negative tests for mismatches between Dart and native types. These new tests do _not yet_ pass on the analyzer. This is tracked in: https://github.com/dart-lang/sdk/issues/49412 TEST=tests/ffi/ffi_native_test.dart TEST=tests/ffi/vmspecific_static_checks_ffinative_test.dart Closes: https://github.com/dart-lang/sdk/issues/49413 Change-Id: I5baded43eab7ff1dc1ffb16550b2a638e4b7a34e Cq-Include-Trybots: luci.dart.try:analyzer-linux-release-try,analyzer-mac-release-try,vm-ffi-android-debug-arm-try,vm-ffi-android-debug-arm64c-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/250843 Reviewed-by: Martin Kustermann <kustermann@google.com> Commit-Queue: Daco Harkes <dacoharkes@google.com>
45 lines
1.4 KiB
Dart
45 lines
1.4 KiB
Dart
// Copyright (c) 2021, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// NOTE: There is no `test/ffi_2/...` version of this test since annotations
|
|
// with type arguments isn't supported in that version of Dart.
|
|
|
|
import 'dart:ffi';
|
|
import 'dart:nativewrappers';
|
|
|
|
class Classy {
|
|
@FfiNative<IntPtr Function(IntPtr)>('ReturnIntPtr')
|
|
external static int returnIntPtrStatic(int x);
|
|
|
|
@FfiNative<Void Function(Handle, IntPtr)>('doesntmatter')
|
|
external void goodHasReceiverHandle(int v);
|
|
}
|
|
|
|
class NativeClassy extends NativeFieldWrapperClass1 {
|
|
@FfiNative<IntPtr Function(IntPtr)>('ReturnIntPtr')
|
|
external static int returnIntPtrStatic(int x);
|
|
|
|
@FfiNative<Void Function(Pointer<Void>, IntPtr)>('doesntmatter')
|
|
external void goodHasReceiverPointer(int v);
|
|
|
|
@FfiNative<Void Function(Handle, IntPtr)>('doesntmatter')
|
|
external void goodHasReceiverHandle(int v);
|
|
}
|
|
|
|
// Regression test: Ensure same-name FfiNative functions don't collide in the
|
|
// top-level namespace, but instead live under their parent (Library, Class).
|
|
class A {
|
|
@FfiNative<Void Function()>('nop')
|
|
external static void foo();
|
|
}
|
|
|
|
class B {
|
|
@FfiNative<Void Function()>('nop')
|
|
external static void foo();
|
|
}
|
|
|
|
void main() {
|
|
/* Intentionally empty: Checks that the transform succeeds. */
|
|
}
|