mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 16:41:07 +00:00
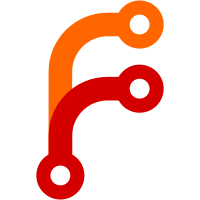
IsolateData contains AppSnapshot which might own some HeapPage-s, so we can't destroy IsolateData in the Dart_IsolateShutdownCallback, because some thread running in the isolate (e.g. background compiler) might still touch thoses pages or objects they host. We need to delay destruction of AppSnapshot until after the isolate shutdown. Current API does not allow that, so we introduce a new isolate lifecycle callback - Dart_IsolateCleanupCallback, which is invoked at the end of the isolote lifecycle when things like background compiler have been stopped and no Dart code is supposed to run. BUG= R=rmacnak@google.com Review-Url: https://codereview.chromium.org/2720723005 .
86 lines
2.1 KiB
C++
86 lines
2.1 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_BIN_ISOLATE_DATA_H_
|
|
#define RUNTIME_BIN_ISOLATE_DATA_H_
|
|
|
|
#include "include/dart_api.h"
|
|
#include "platform/assert.h"
|
|
#include "platform/globals.h"
|
|
|
|
namespace dart {
|
|
|
|
// Forward declaration.
|
|
template <typename T>
|
|
class MallocGrowableArray;
|
|
|
|
} // namespace dart
|
|
|
|
namespace dart {
|
|
namespace bin {
|
|
|
|
// Forward declaration.
|
|
class AppSnapshot;
|
|
class EventHandler;
|
|
class Loader;
|
|
|
|
// Data associated with every isolate in the standalone VM
|
|
// embedding. This is used to free external resources for each isolate
|
|
// when the isolate shuts down.
|
|
class IsolateData {
|
|
public:
|
|
IsolateData(const char* url,
|
|
const char* package_root,
|
|
const char* packages_file,
|
|
AppSnapshot* app_snapshot);
|
|
~IsolateData();
|
|
|
|
Dart_Handle builtin_lib() const {
|
|
ASSERT(builtin_lib_ != NULL);
|
|
ASSERT(!Dart_IsError(builtin_lib_));
|
|
return builtin_lib_;
|
|
}
|
|
void set_builtin_lib(Dart_Handle lib) {
|
|
ASSERT(builtin_lib_ == NULL);
|
|
ASSERT(lib != NULL);
|
|
ASSERT(!Dart_IsError(lib));
|
|
builtin_lib_ = Dart_NewPersistentHandle(lib);
|
|
}
|
|
|
|
char* script_url;
|
|
char* package_root;
|
|
char* packages_file;
|
|
uint8_t* udp_receive_buffer;
|
|
|
|
// While loading a loader is associated with the isolate.
|
|
bool HasLoader() const { return loader_ != NULL; }
|
|
Loader* loader() const {
|
|
ASSERT(loader_ != NULL);
|
|
return loader_;
|
|
}
|
|
void set_loader(Loader* loader) {
|
|
ASSERT((loader_ == NULL) || (loader == NULL));
|
|
loader_ = loader;
|
|
}
|
|
MallocGrowableArray<char*>* dependencies() const { return dependencies_; }
|
|
void set_dependencies(MallocGrowableArray<char*>* deps) {
|
|
dependencies_ = deps;
|
|
}
|
|
|
|
void OnIsolateShutdown();
|
|
|
|
private:
|
|
Dart_Handle builtin_lib_;
|
|
Loader* loader_;
|
|
AppSnapshot* app_snapshot_;
|
|
MallocGrowableArray<char*>* dependencies_;
|
|
|
|
DISALLOW_COPY_AND_ASSIGN(IsolateData);
|
|
};
|
|
|
|
} // namespace bin
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_BIN_ISOLATE_DATA_H_
|