mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 04:27:17 +00:00
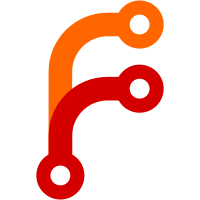
This reverts commitc97f7b7fad
. Reason for revert: Still breaking google3 (b/236665701) Original change's description: > Make `nullFuture` be per-zone. > > We introduced a `nullFuture` during the null-safety migration where > we changed some methods to no longer allow returning `null`, > and they therefore had to return a `Future`. > That affected timing, because returning `null` was processed > synchronously, and that change in timing made some tests fail. > Rather that fix the fragile tests, we made the function return > a recognizable future, a canonical `Future<Null>.value(null)`, > and then recognized it and took a synchronous path for it. > > That caused other issues, because the future was created in the > root zone. (Well, originally, it was created in the first zone > which needed one, that was worse. Now it's created in the root zone.) > Some code tries to contain asynchrony inside a custom zone, and > then the get a `nullFuture` and calls `then` on it, and that > schedules a microtask in the root zone. > (It should probably have used the listener's zone, and not store > a zone in the future at all, but that's how it was first done, > and now people rely on that behavior too.) > > This change creates a `null` future *per zone* (lazily initialized > when asked for). That should be sufficient because the code recognizing > a returned `null` future is generally running in the same zone, > but if any other code gets the `nullFuture`, it will be in the > expected zone for where it was requested. > > This is a reland of commita247b158d6
> > Change-Id: Ieec74d6f93c57175c357ec18889144635f5bdca6 > Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/249490 > Commit-Queue: Lasse Nielsen <lrn@google.com> > Reviewed-by: Erik Ernst <eernst@google.com> TBR=lrn@google.com,eernst@google.com,nbosch@google.com Change-Id: I870285b03ec05803c5aaa6b66f9a6e6ea360d732 No-Presubmit: true No-Tree-Checks: true No-Try: true Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/249609 Reviewed-by: Lasse Nielsen <lrn@google.com> Commit-Queue: Emmanuel Pellereau <emmanuelp@google.com> Reviewed-by: Emmanuel Pellereau <emmanuelp@google.com>
37 lines
1,023 B
Dart
37 lines
1,023 B
Dart
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import "package:expect/expect.dart";
|
|
import "package:async_helper/async_helper.dart";
|
|
import 'dart:async';
|
|
|
|
main() {
|
|
asyncStart(2);
|
|
() async {
|
|
var it = new StreamIterator(new Stream.fromIterable([]));
|
|
Expect.isFalse(await it.moveNext());
|
|
|
|
late Future nullFuture;
|
|
late Future falseFuture;
|
|
|
|
runZoned(() {
|
|
nullFuture = (new StreamController()..stream.listen(null).cancel()).done;
|
|
falseFuture = it.moveNext();
|
|
}, zoneSpecification: new ZoneSpecification(scheduleMicrotask:
|
|
(Zone self, ZoneDelegate parent, Zone zone, void f()) {
|
|
Expect.fail("Should not be called");
|
|
}));
|
|
|
|
nullFuture.then((value) {
|
|
Expect.isNull(value);
|
|
asyncEnd();
|
|
});
|
|
|
|
falseFuture.then((value) {
|
|
Expect.isFalse(value);
|
|
asyncEnd();
|
|
});
|
|
}();
|
|
}
|