mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 04:16:51 +00:00
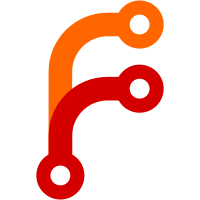
This changes the span reported from the _receiver_ to the _use_ (method name, property name, operator token). I think this change is overall an improvement. Specifically, its a great improvement for cmdline output, where the receiver and the "use" are on different lines. One possibly weird change is that if the operator is `[]`, then I only highlight the `[` character. I don't know if there is a better place, and I think this is fine. Fixes https://github.com/dart-lang/sdk/issues/43708 Change-Id: Ie66ddf04b4904a367575193106385dd63ee39985 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/188680 Commit-Queue: Samuel Rawlins <srawlins@google.com> Reviewed-by: Konstantin Shcheglov <scheglov@google.com> Reviewed-by: Brian Wilkerson <brianwilkerson@google.com>
56 lines
1.4 KiB
Dart
56 lines
1.4 KiB
Dart
// Copyright (c) 2020, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// This test verifies that attempts to promote the type of `this` inside an
|
|
// extension method have no effect.
|
|
|
|
void f(dynamic d) {}
|
|
|
|
class C {
|
|
int? cProp;
|
|
}
|
|
|
|
extension on C? {
|
|
void testCQuestion() {
|
|
if (this != null) {
|
|
f(this.cProp);
|
|
// ^^^^^
|
|
// [analyzer] COMPILE_TIME_ERROR.UNCHECKED_USE_OF_NULLABLE_VALUE
|
|
// ^
|
|
// [cfe] Property 'cProp' cannot be accessed on 'C?' because it is potentially null.
|
|
f(cProp);
|
|
//^^^^^
|
|
// [analyzer] COMPILE_TIME_ERROR.UNCHECKED_USE_OF_NULLABLE_VALUE
|
|
// [cfe] Property 'cProp' cannot be accessed on 'C?' because it is potentially null.
|
|
}
|
|
}
|
|
}
|
|
|
|
class D extends C {
|
|
int? dProp;
|
|
}
|
|
|
|
extension on C {
|
|
void testC() {
|
|
if (this is D) {
|
|
f(this.dProp);
|
|
// ^^^^^
|
|
// [analyzer] COMPILE_TIME_ERROR.UNDEFINED_GETTER
|
|
// [cfe] The getter 'dProp' isn't defined for the class 'C'.
|
|
f(dProp);
|
|
//^^^^^
|
|
// [analyzer] COMPILE_TIME_ERROR.UNDEFINED_IDENTIFIER
|
|
// [cfe] The getter 'dProp' isn't defined for the class 'C'.
|
|
}
|
|
}
|
|
}
|
|
|
|
main() {
|
|
C().testCQuestion();
|
|
C().testC();
|
|
D().testCQuestion();
|
|
D().testC();
|
|
(null as C?).testCQuestion();
|
|
}
|