mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 16:41:07 +00:00
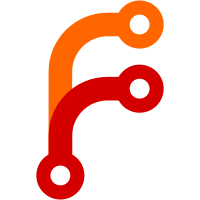
This CL implements `NativeFinalizer` in the GC. `FinalizerEntry`s are extended to track `external_size` and in which `Heap::Space` the finalizable value is. On attaching a native finalizer, the external size is added to the relevant heap. When the finalizable value is promoted from new to old space, the external size is promoted as well. And when a native finalizer is run or is detached, the external size is removed from the relevant heap again. In contrast to Dart `Finalizer`s, `NativeFinalizer`s are run on isolate shutdown. When the `NativeFinalizer`s themselves are collected, the finalizers are not run. Users should stick the native finalizer in a global variable to ensure finalization. We will revisit this design when we add send and exit support, because there is a design space to explore what to do in that case. This current solution promises the least to users. In this implementation native finalizers have a Dart entry to clean up the entries from the `all_entries` field of the finalizer. We should consider using another data structure that avoids the need for this Dart entry. See the TODO left in the code. Bug: https://github.com/dart-lang/sdk/issues/47777 TEST=runtime/tests/vm/dart(_2)/isolates/fast_object_copy_test.dart TEST=runtime/vm/object_test.cc TEST=tests/ffi(_2)/vmspecific_native_finalizer_* Change-Id: I8f594c80c3c344ad83e1f2de10de028eb8456121 Cq-Include-Trybots: luci.dart.try:vm-kernel-reload-rollback-linux-debug-x64-try,vm-kernel-reload-linux-debug-x64-try,vm-ffi-android-debug-arm64c-try,dart-sdk-mac-arm64-try,vm-kernel-mac-release-arm64-try,pkg-mac-release-arm64-try,vm-kernel-precomp-nnbd-mac-release-arm64-try,vm-kernel-win-debug-x64c-try,vm-kernel-win-debug-x64-try,vm-kernel-precomp-win-debug-x64c-try,vm-kernel-nnbd-win-release-ia32-try,vm-ffi-android-debug-arm-try,vm-precomp-ffi-qemu-linux-release-arm-try,vm-kernel-mac-debug-x64-try,vm-kernel-nnbd-mac-debug-x64-try,vm-kernel-nnbd-linux-debug-ia32-try,benchmark-linux-try,flutter-frontend-try,pkg-linux-debug-try,vm-kernel-asan-linux-release-x64-try,vm-kernel-gcc-linux-try,vm-kernel-optcounter-threshold-linux-release-x64-try,vm-kernel-precomp-linux-debug-simarm_x64-try,vm-kernel-precomp-obfuscate-linux-release-x64-try,vm-kernel-precomp-linux-debug-x64-try,vm-kernel-precomp-linux-debug-x64c-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/236320 Reviewed-by: Martin Kustermann <kustermann@google.com> Reviewed-by: Slava Egorov <vegorov@google.com> Commit-Queue: Daco Harkes <dacoharkes@google.com>
66 lines
1.9 KiB
Dart
66 lines
1.9 KiB
Dart
// Copyright (c) 2019, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
//
|
|
// Helpers for tests which trigger GC in delicate places.
|
|
|
|
// @dart = 2.9
|
|
|
|
// ignore: import_internal_library, unused_import
|
|
import 'dart:_internal';
|
|
import 'dart:ffi';
|
|
|
|
import 'dylib_utils.dart';
|
|
|
|
typedef NativeNullaryOp = Void Function();
|
|
typedef NullaryOpVoid = void Function();
|
|
|
|
typedef NativeUnaryOp = Void Function(IntPtr);
|
|
typedef UnaryOpVoid = void Function(int);
|
|
|
|
final DynamicLibrary ffiTestFunctions =
|
|
dlopenPlatformSpecific("ffi_test_functions");
|
|
|
|
final collectOnNthAllocation = ffiTestFunctions
|
|
.lookupFunction<NativeUnaryOp, UnaryOpVoid>("CollectOnNthAllocation");
|
|
|
|
extension PointerOffsetBy<T extends NativeType> on Pointer<T> {
|
|
Pointer<T> offsetBy(int bytes) => Pointer.fromAddress(address + bytes);
|
|
}
|
|
|
|
/// Triggers garbage collection.
|
|
// Defined in `dart:_internal`.
|
|
// ignore: undefined_identifier
|
|
void triggerGc() => VMInternalsForTesting.collectAllGarbage();
|
|
|
|
void Function(String) _namedPrint(String name) {
|
|
if (name != null) {
|
|
return (String value) => print('$name: $value');
|
|
}
|
|
return (String value) => print(value);
|
|
}
|
|
|
|
/// Does a GC and if [doAwait] awaits a future to enable running finalizers.
|
|
///
|
|
/// Also prints for debug purposes.
|
|
///
|
|
/// If provided, [name] prefixes the debug prints.
|
|
void doGC({String name}) {
|
|
final _print = _namedPrint(name);
|
|
|
|
_print('Do GC.');
|
|
triggerGc();
|
|
_print('GC done');
|
|
}
|
|
|
|
void createAndLoseFinalizable(Pointer<IntPtr> token) {
|
|
final myFinalizable = MyFinalizable();
|
|
setTokenFinalizer.attach(myFinalizable, token.cast());
|
|
}
|
|
|
|
final setTokenTo42Ptr =
|
|
ffiTestFunctions.lookup<NativeFinalizerFunction>("SetArgumentTo42");
|
|
final setTokenFinalizer = NativeFinalizer(setTokenTo42Ptr);
|
|
|
|
class MyFinalizable implements Finalizable {}
|