mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 01:45:06 +00:00
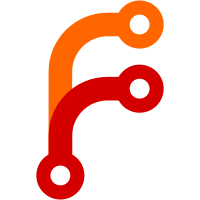
Previously repetetively parsing some string valued flags would cause their values to leak. This change makes sure that we delete the previous value when we assign a new one. vm/cc/ParseFlags is extended to catch this when running under ASAN. TEST=tools/test.py -n dartk-asan-linux-release-x64 vm/cc/ParseFlags Change-Id: I7478cdb48063dcae35d4129a4c9a2829dddae729 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/267821 Reviewed-by: Ryan Macnak <rmacnak@google.com> Commit-Queue: Slava Egorov <vegorov@google.com>
58 lines
2 KiB
C++
58 lines
2 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#include "vm/flags.h"
|
|
#include "platform/assert.h"
|
|
#include "vm/heap/heap.h"
|
|
#include "vm/unit_test.h"
|
|
|
|
namespace dart {
|
|
|
|
DEFINE_FLAG(bool, basic_flag, true, "Testing of a basic boolean flag.");
|
|
|
|
DECLARE_FLAG(bool, print_flags);
|
|
|
|
VM_UNIT_TEST_CASE(BasicFlags) {
|
|
EXPECT_EQ(true, FLAG_basic_flag);
|
|
EXPECT_EQ(false, FLAG_verbose_gc);
|
|
EXPECT_EQ(false, FLAG_print_flags);
|
|
}
|
|
|
|
DEFINE_FLAG(bool, parse_flag_bool_test, true, "Flags::Parse (bool) testing");
|
|
DEFINE_FLAG(charp, string_opt_test, NULL, "Testing: string option.");
|
|
DEFINE_FLAG(charp, entrypoint_test, "main", "Testing: entrypoint");
|
|
DEFINE_FLAG(int, counter, 100, "Testing: int flag");
|
|
|
|
VM_UNIT_TEST_CASE(ParseFlags) {
|
|
EXPECT_EQ(true, FLAG_parse_flag_bool_test);
|
|
Flags::Parse("no_parse_flag_bool_test");
|
|
EXPECT_EQ(false, FLAG_parse_flag_bool_test);
|
|
Flags::Parse("parse_flag_bool_test");
|
|
EXPECT_EQ(true, FLAG_parse_flag_bool_test);
|
|
Flags::Parse("parse_flag_bool_test=false");
|
|
EXPECT_EQ(false, FLAG_parse_flag_bool_test);
|
|
Flags::Parse("parse_flag_bool_test=true");
|
|
EXPECT_EQ(true, FLAG_parse_flag_bool_test);
|
|
|
|
EXPECT_EQ(true, FLAG_string_opt_test == NULL);
|
|
Flags::Parse("string_opt_test=doobidoo");
|
|
EXPECT_EQ(true, FLAG_string_opt_test != NULL);
|
|
EXPECT_EQ(0, strcmp(FLAG_string_opt_test, "doobidoo"));
|
|
FLAG_string_opt_test = reinterpret_cast<charp>(0xDEADBEEF);
|
|
Flags::Parse("string_opt_test=foofoo");
|
|
EXPECT_EQ(true, FLAG_string_opt_test != NULL);
|
|
EXPECT_EQ(0, strcmp(FLAG_string_opt_test, "foofoo"));
|
|
|
|
EXPECT_EQ(true, FLAG_entrypoint_test != NULL);
|
|
EXPECT_EQ(0, strcmp(FLAG_entrypoint_test, "main"));
|
|
|
|
EXPECT_EQ(100, FLAG_counter);
|
|
Flags::Parse("counter=-300");
|
|
EXPECT_EQ(-300, FLAG_counter);
|
|
Flags::Parse("counter=$300");
|
|
EXPECT_EQ(-300, FLAG_counter);
|
|
}
|
|
|
|
} // namespace dart
|