mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 03:07:49 +00:00
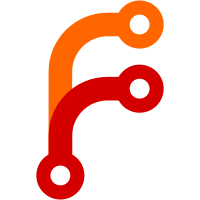
This will automatically desugar FfiNative calls passing NativeFieldWrapperClass1 handles to use Pointer when the underlying native function expects a pointer. E.g.: ``` class ClassWithNativeField extends NativeFieldWrapperClass1 {} @FfiNative<IntPtr Function(Pointer<Void>)>('PassAsPointer') external int passAsPointer(ClassWithNativeField obj); passAsPointer(ClassWithNativeField()); // Becomes roughly: // #t0 = PointerClassWithNativeField(); // passAsPointer(Pointer.fromAddress(getNativeField(#t0))); // reachabilityFence(#t0); ``` TEST=Adds new tests in tests/ffi/ffi_native_test.dart. Change-Id: I4460f9249803054f12be5d5ff34dbdf7c96567fd Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/213260 Commit-Queue: Clement Skau <cskau@google.com> Reviewed-by: Daco Harkes <dacoharkes@google.com>
42 lines
1.5 KiB
Dart
42 lines
1.5 KiB
Dart
// Copyright (c) 2021, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// NOTE: There is no `test/ffi_2/...` version of this test since annotations
|
|
// with type arguments isn't supported in that version of Dart.
|
|
|
|
import 'dart:ffi';
|
|
import 'dart:nativewrappers';
|
|
|
|
import 'package:expect/expect.dart';
|
|
|
|
// Error: FFI leaf call must not have Handle return type.
|
|
@FfiNative<Handle Function()>("foo", isLeaf: true) //# 01: compile-time error
|
|
external Object foo(); //# 01: compile-time error
|
|
|
|
// Error: FFI leaf call must not have Handle argument types.
|
|
@FfiNative<Void Function(Handle)>("bar", isLeaf: true) //# 02: compile-time error
|
|
external void bar(Object); //# 02: compile-time error
|
|
|
|
class Classy {
|
|
@FfiNative<IntPtr Function(IntPtr)>('ReturnIntPtr')
|
|
external static int returnIntPtrStatic(int x);
|
|
|
|
// Error: FfiNative annotations can only be used on static functions.
|
|
@FfiNative<IntPtr Function(IntPtr)>('ReturnIntPtr') //# 03: compile-time error
|
|
external int returnIntPtrMethod(int x); //# 03: compile-time error
|
|
}
|
|
|
|
// Regression test: Ensure same-name FfiNative functions don't collide in the
|
|
// top-level namespace, but instead live under their parent (Library, Class).
|
|
class A {
|
|
@FfiNative<Void Function()>('nop')
|
|
external static void foo();
|
|
}
|
|
class B {
|
|
@FfiNative<Void Function()>('nop')
|
|
external static void foo();
|
|
}
|
|
|
|
void main() { /* Intentionally empty: Compile-time error tests. */ }
|