mirror of
https://github.com/dart-lang/sdk
synced 2024-10-03 16:26:40 +00:00
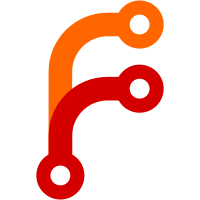
Running trust evaluation system api call on worker thread effectively unblocks main isolate when it attempts to establish https connection. Execution of the worker thread is implemented via dart native port infrastructure which allows to run C++ code as a dart message handler. TrustEvaluateHandlerFunc (if provided by platform-dependent SSLCertContext) is that handler, only mac(ios) implementation provides it. Asynchrony of CertificateVerificationCallback (for mac/ios) is implemented via [ssl_verify_retry] return code which allows to suspend ssl handshake until certificate trust is confirmed. When dart's _RawSecureSocket.secureHandshake() method that initiated ssl's handshake received this return code it knows it has to wait for a future that is completed by another callback([rpEvaluteResponse]) that waits for trust evaluation handler response. rpEvaluateResponse purpose is to listen for response from TrustEvaluateHandler and also invoke user-provided [badCertficateCallback] that can override trust decision for a given connection request, for a given certificate. Once that future is completed and CertificateVerificationCallback knows whether to trust a certificate or not, _secureHandshake retries ssl handshake. Bug: https://github.com/dart-lang/sdk/issues/41519 Change-Id: Ifee18639c78099ec77cad50000444bc6c7b9369b Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/165520 Reviewed-by: Siva Annamalai <asiva@google.com> Commit-Queue: Alexander Aprelev <aam@google.com>
187 lines
6.3 KiB
C++
187 lines
6.3 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#if defined(DART_IO_SECURE_SOCKET_DISABLED)
|
|
|
|
#include "bin/builtin.h"
|
|
#include "bin/dartutils.h"
|
|
#include "include/dart_api.h"
|
|
|
|
namespace dart {
|
|
namespace bin {
|
|
|
|
void FUNCTION_NAME(SecureSocket_Init)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_Connect)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_AddCertificate)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_Destroy)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_Handshake)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_MarkAsTrusted)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_NewX509CertificateWrapper)(
|
|
Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_GetSelectedProtocol)(
|
|
Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_RegisterHandshakeCompleteCallback)(
|
|
Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_RegisterBadCertificateCallback)(
|
|
Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_ProcessBuffer)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_InitializeLibrary)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_PeerCertificate)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_FilterPointer)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_Renegotiate)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecureSocket_NewServicePort)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecurityContext_Allocate)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecurityContext_UsePrivateKeyBytes)(
|
|
Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecurityContext_SetAlpnProtocols)(
|
|
Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecurityContext_SetClientAuthoritiesBytes)(
|
|
Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecurityContext_SetTrustedCertificatesBytes)(
|
|
Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecurityContext_TrustBuiltinRoots)(
|
|
Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(SecurityContext_UseCertificateChainBytes)(
|
|
Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(X509_Der)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(X509_Sha1)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(X509_Subject)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(X509_Issuer)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(X509_StartValidity)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(X509_EndValidity)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
void FUNCTION_NAME(X509_Pem)(Dart_NativeArguments args) {
|
|
Dart_ThrowException(DartUtils::NewDartArgumentError(
|
|
"Secure Sockets unsupported on this platform"));
|
|
}
|
|
|
|
class SSLFilter {
|
|
public:
|
|
static CObject* ProcessFilterRequest(const CObjectArray& request);
|
|
};
|
|
|
|
CObject* SSLFilter::ProcessFilterRequest(const CObjectArray& request) {
|
|
return CObject::IllegalArgumentError();
|
|
}
|
|
|
|
} // namespace bin
|
|
} // namespace dart
|
|
|
|
#endif // defined(DART_IO_SECURE_SOCKET_DISABLED)
|