mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
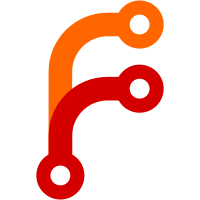
* Migrate to python3; drop python support. * Update Windows toolchain support. * Remove some unused methods. * Python 2.7 is still needed on Windows. * Update gsutil to a version that supports python3. Fixes: https://github.com/dart-lang/sdk/issues/28793 TEST=Manually tested common user journeys. Change-Id: I663a22b237a548bb82dc2e601e399e3bc3649211 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/192182 Reviewed-by: William Hesse <whesse@google.com> Reviewed-by: Alexander Aprelev <aam@google.com>
57 lines
1.9 KiB
Python
Executable file
57 lines
1.9 KiB
Python
Executable file
#!/usr/bin/env python3
|
|
# Copyright (c) 2011, the Dart project authors. Please see the AUTHORS file
|
|
# for details. All rights reserved. Use of this source code is governed by a
|
|
# BSD-style license that can be found in the LICENSE file.
|
|
|
|
from contextlib import ExitStack
|
|
import os
|
|
import string
|
|
import subprocess
|
|
import sys
|
|
|
|
import utils
|
|
|
|
|
|
def Main():
|
|
args = sys.argv[1:]
|
|
|
|
cleanup_dart = False
|
|
if '--cleanup-dart-processes' in args:
|
|
args.remove('--cleanup-dart-processes')
|
|
cleanup_dart = True
|
|
|
|
tools_dir = os.path.dirname(os.path.realpath(__file__))
|
|
repo_dir = os.path.dirname(tools_dir)
|
|
dart_test_script = os.path.join(repo_dir, 'pkg', 'test_runner', 'bin',
|
|
'test_runner.dart')
|
|
command = [utils.CheckedInSdkExecutable(), dart_test_script] + args
|
|
|
|
# The testing script potentially needs the android platform tools in PATH so
|
|
# we do that in ./tools/test.py (a similar logic exists in ./tools/build.py).
|
|
android_platform_tools = os.path.normpath(
|
|
os.path.join(tools_dir,
|
|
'../third_party/android_tools/sdk/platform-tools'))
|
|
if os.path.isdir(android_platform_tools):
|
|
os.environ['PATH'] = '%s%s%s' % (os.environ['PATH'], os.pathsep,
|
|
android_platform_tools)
|
|
|
|
with utils.FileDescriptorLimitIncreaser():
|
|
with ExitStack() as stack:
|
|
for ctx in utils.CoreDumpArchiver(args):
|
|
stack.enter_context(ctx)
|
|
exit_code = subprocess.call(command)
|
|
|
|
if cleanup_dart:
|
|
cleanup_command = [
|
|
sys.executable,
|
|
os.path.join(tools_dir, 'task_kill.py'), '--kill_dart=True',
|
|
'--kill_vc=False'
|
|
]
|
|
subprocess.call(cleanup_command)
|
|
|
|
utils.DiagnoseExitCode(exit_code, command)
|
|
return exit_code
|
|
|
|
|
|
if __name__ == '__main__':
|
|
sys.exit(Main())
|