mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 16:41:07 +00:00
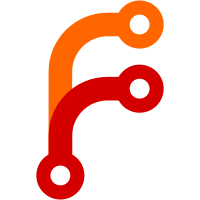
While any error listener is attached to an isolate, uncaught errors are reported to the listener through his supplied sendPort. The current format for the error is [error.toString(), stack == null ? null : stack.toString()] This is wrapped on the receiving side. The isolate exposes the errors as a broadcast stream of error events. R=floitsch@google.com Review URL: https://codereview.chromium.org//169703002 git-svn-id: https://dart.googlecode.com/svn/branches/bleeding_edge/dart@36290 260f80e4-7a28-3924-810f-c04153c831b5
72 lines
2 KiB
Dart
72 lines
2 KiB
Dart
// Copyright (c) 2014, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
library handle_error_test;
|
|
|
|
import "dart:isolate";
|
|
import "dart:async";
|
|
import "package:async_helper/async_helper.dart";
|
|
import "package:expect/expect.dart";
|
|
|
|
isomain1(replyPort) {
|
|
RawReceivePort port = new RawReceivePort();
|
|
port.handler = (v) {
|
|
switch (v) {
|
|
case 0:
|
|
replyPort.send(42);
|
|
break;
|
|
case 1:
|
|
throw new ArgumentError("whoops");
|
|
case 2:
|
|
throw new RangeError.value(37);
|
|
case 3:
|
|
port.close();
|
|
}
|
|
};
|
|
replyPort.send(port.sendPort);
|
|
}
|
|
|
|
main(){
|
|
asyncStart();
|
|
RawReceivePort reply = new RawReceivePort(null);
|
|
// Start paused so we have time to set up the error handler.
|
|
Isolate.spawn(isomain1, reply.sendPort, paused: true).then((Isolate isolate) {
|
|
isolate.setErrorsFatal(false);
|
|
Stream errors = isolate.errors; // Broadcast stream, never a done message.
|
|
SendPort sendPort;
|
|
StreamSubscription subscription;
|
|
int state = 0;
|
|
reply.handler = (port) {
|
|
sendPort = port;
|
|
port.send(state);
|
|
reply.handler = (v) {
|
|
Expect.equals(0, state);
|
|
Expect.equals(42, v);
|
|
state++;
|
|
sendPort.send(state);
|
|
};
|
|
};
|
|
subscription = errors.listen(null, onError: (error, stack) {
|
|
switch (state) {
|
|
case 1:
|
|
Expect.equals(new ArgumentError("whoops").toString(), "$error");
|
|
state++;
|
|
sendPort.send(state);
|
|
break;
|
|
case 2:
|
|
Expect.equals(new RangeError.value(37).toString(), "$error");
|
|
state++;
|
|
sendPort.send(state);
|
|
reply.close();
|
|
subscription.cancel();
|
|
asyncEnd();
|
|
break;
|
|
default:
|
|
throw "Bad state for error: $state: $error";
|
|
}
|
|
});
|
|
isolate.resume(isolate.pauseCapability);
|
|
});
|
|
}
|