mirror of
https://github.com/dart-lang/sdk
synced 2024-09-18 21:51:18 +00:00
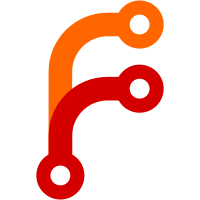
Some artifacts are .exe instead of .bat. Library uris with file schemes use the Uri.path (using forward slashes on Windows), so the tests emitting native asset mappings should use Uri.path as well. (Not Uri.toFilePath() which uses backwards slashes.) The paths of the dynamic libraries in the assets should use the toFilePath(), as these are passed to the system API which expects correct paths. The Platform.script Uri has a path with file:///C: and back slashes. The uri RFC does not support back slashes, and our ResolveUri does neither. So for Windows we replace backslashes with forward slashes. TEST=tests/ffi/native_assets/ Bug: https://github.com/dart-lang/sdk/issues/51066 Bug: https://github.com/dart-lang/sdk/issues/51067 Change-Id: I2e168e0549fe80d9a5366d636c6f1ef971942130 Cq-Include-Trybots: luci.dart.try:vm-kernel-precomp-nnbd-win-release-x64-try,vm-kernel-nnbd-win-debug-x64-try,vm-kernel-nnbd-win-release-x64-try,vm-kernel-nnbd-win-release-ia32-try,vm-kernel-msvc-windows-try,dart-sdk-win-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/279356 Reviewed-by: Martin Kustermann <kustermann@google.com> Commit-Queue: Daco Harkes <dacoharkes@google.com>
112 lines
3 KiB
Dart
112 lines
3 KiB
Dart
// Copyright (c) 2023, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// This test compiles itself with gen_kernel and invokes the compiled kernel
|
|
// file with `Process.run(dart, <...>)` and `Isolate.spawn` and
|
|
// `Isolate.spawnUri`.
|
|
//
|
|
// This tests test including a native asset mapping that looks up its symbols
|
|
// in the process.
|
|
|
|
// OtherResources=asset_process_test.dart
|
|
// OtherResources=helpers.dart
|
|
|
|
// ignore_for_file: deprecated_member_use
|
|
|
|
import 'dart:async';
|
|
import 'dart:ffi';
|
|
import 'dart:io';
|
|
|
|
import 'package:expect/expect.dart';
|
|
|
|
import 'helpers.dart';
|
|
|
|
const runTestsArg = 'run-tests';
|
|
|
|
main(List<String> args, Object? message) async {
|
|
return await selfInvokingTest(
|
|
doOnOuterInvocation: selfInvokes,
|
|
doOnProcessInvocation: () async {
|
|
await runTests();
|
|
await testIsolateSpawn(runTests);
|
|
await testIsolateSpawnUri(spawnUri: Platform.script, arguments: args);
|
|
},
|
|
doOnSpawnUriInvocation: () async {
|
|
await runTests();
|
|
await testIsolateSpawn(runTests);
|
|
},
|
|
)(args, message);
|
|
}
|
|
|
|
const asset2Name = 'myAsset';
|
|
|
|
Future<void> selfInvokes() async {
|
|
final selfSourceUri = Platform.script.resolve('asset_process_test.dart');
|
|
final nativeAssetsYaml = createNativeAssetYaml(
|
|
asset: selfSourceUri.toString(),
|
|
assetMapping: ['process'],
|
|
asset2: asset2Name,
|
|
asset2Mapping: ['process'],
|
|
);
|
|
await invokeSelf(
|
|
selfSourceUri: selfSourceUri,
|
|
runtime: Runtime.jit,
|
|
arguments: [runTestsArg],
|
|
nativeAssetsYaml: nativeAssetsYaml,
|
|
);
|
|
await invokeSelf(
|
|
selfSourceUri: selfSourceUri,
|
|
runtime: Runtime.aot,
|
|
arguments: [runTestsArg],
|
|
nativeAssetsYaml: nativeAssetsYaml,
|
|
);
|
|
}
|
|
|
|
Future<void> runTests() async {
|
|
testProcessOrSystem();
|
|
testNonExistingFunction();
|
|
}
|
|
|
|
@FfiNative<Pointer Function(IntPtr)>('malloc')
|
|
external Pointer posixMalloc(int size);
|
|
|
|
@FfiNative<Void Function(Pointer)>('free')
|
|
external void posixFree(Pointer pointer);
|
|
|
|
@FfiNative<Pointer Function(Size)>('CoTaskMemAlloc')
|
|
external Pointer winCoTaskMemAlloc(int cb);
|
|
|
|
@FfiNative<Void Function(Pointer)>('CoTaskMemFree')
|
|
external void winCoTaskMemFree(Pointer pv);
|
|
|
|
@Native<Pointer Function(IntPtr)>()
|
|
external Pointer malloc(int size);
|
|
|
|
@Native<Void Function(Pointer)>(assetId: asset2Name)
|
|
external void free(Pointer pointer);
|
|
|
|
@Native<Pointer Function(Size)>()
|
|
external Pointer CoTaskMemAlloc(int cb);
|
|
|
|
@Native<Void Function(Pointer)>(assetId: asset2Name)
|
|
external void CoTaskMemFree(Pointer pv);
|
|
|
|
void testProcessOrSystem() {
|
|
if (Platform.isWindows) {
|
|
final pointer = winCoTaskMemAlloc(8);
|
|
Expect.notEquals(nullptr, pointer);
|
|
winCoTaskMemFree(pointer);
|
|
final pointer2 = CoTaskMemAlloc(8);
|
|
Expect.notEquals(nullptr, pointer2);
|
|
CoTaskMemFree(pointer2);
|
|
} else {
|
|
final pointer = posixMalloc(8);
|
|
Expect.notEquals(nullptr, pointer);
|
|
posixFree(pointer);
|
|
final pointer2 = malloc(8);
|
|
Expect.notEquals(nullptr, pointer2);
|
|
free(pointer2);
|
|
}
|
|
}
|