mirror of
https://github.com/dart-lang/sdk
synced 2024-09-20 04:31:31 +00:00
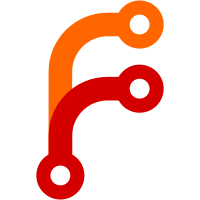
This includes support for calling Dart_PropagateError in native code when doing FFI calls, and catching uncaught exceptions with Dart_IsError when doing FFI callbacks. The support for Dart_PropagateError adds a catch entry to the FFI trampoline, which prevents inlining these trampolines in AOT. This regresses the FfiCall benchmarks by 1-2% in AOT. In addition, Dart_PropagateError requires maintaining a bit whether we entered native/VM code from generated code through FFI or not. That way we can do the proper transition on the exception path. When entering generated code, we store this bit on the stack, right after the entry frame. Design: http://go/dart-ffi-handles Issue: https://github.com/dart-lang/sdk/issues/36858 Issue: https://github.com/dart-lang/sdk/issues/41319 Change-Id: Idfd7ff69132fb29cc730931a4113d914d4437396 Cq-Include-Trybots: luci.dart.try:vm-ffi-android-debug-arm-try,vm-ffi-android-debug-arm64-try,app-kernel-linux-debug-x64-try,vm-kernel-linux-debug-ia32-try,vm-kernel-win-debug-x64-try,vm-kernel-win-debug-ia32-try,vm-kernel-precomp-linux-debug-x64-try,vm-dartkb-linux-release-x64-abi-try,vm-kernel-precomp-android-release-arm64-try,vm-kernel-asan-linux-release-x64-try,vm-kernel-linux-release-simarm-try,vm-kernel-linux-release-simarm64-try,vm-kernel-precomp-android-release-arm_x64-try,vm-kernel-precomp-obfuscate-linux-release-x64-try,dart-sdk-linux-try,analyzer-analysis-server-linux-try,analyzer-linux-release-try,front-end-linux-release-x64-try,vm-kernel-precomp-win-release-x64-try,vm-kernel-mac-debug-x64-try,vm-precomp-ffi-qemu-linux-release-arm-try,vm-kernel-nnbd-linux-debug-x64-try,analyzer-nnbd-linux-release-try,front-end-nnbd-linux-release-x64-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/145591 Commit-Queue: Daco Harkes <dacoharkes@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com> Reviewed-by: Alexander Markov <alexmarkov@google.com>
74 lines
2.8 KiB
C++
74 lines
2.8 KiB
C++
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_STACK_FRAME_ARM_H_
|
|
#define RUNTIME_VM_STACK_FRAME_ARM_H_
|
|
|
|
#if !defined(RUNTIME_VM_STACK_FRAME_H_)
|
|
#error Do not include stack_frame_arm.h directly; use stack_frame.h instead.
|
|
#endif
|
|
|
|
namespace dart {
|
|
|
|
/* ARM Dart Frame Layout
|
|
|
|
| | <- TOS
|
|
Callee frame | ... |
|
|
| saved PP | (PP of current frame)
|
|
| code object |
|
|
| saved FP | (FP of current frame)
|
|
| saved LR | (PC of current frame)
|
|
+--------------------+
|
|
Current frame | ... T| <- SP of current frame
|
|
| first local T|
|
|
| caller's PP T|
|
|
| code object T| (current frame's code object)
|
|
| caller's FP | <- FP of current frame
|
|
| caller's LR | (PC of caller frame)
|
|
+--------------------+
|
|
Caller frame | last parameter | <- SP of caller frame
|
|
| ... |
|
|
|
|
T against a slot indicates it needs to be traversed during GC.
|
|
*/
|
|
|
|
static const int kDartFrameFixedSize = 4; // PP, FP, LR, PC marker.
|
|
static const int kSavedPcSlotFromSp = -1;
|
|
|
|
static const int kFirstObjectSlotFromFp = -1; // Used by GC to traverse stack.
|
|
static const int kLastFixedObjectSlotFromFp = -2;
|
|
|
|
static const int kFirstLocalSlotFromFp = -3;
|
|
static const int kSavedCallerPpSlotFromFp = -2;
|
|
static const int kPcMarkerSlotFromFp = -1;
|
|
static const int kSavedCallerFpSlotFromFp = 0;
|
|
static const int kSavedCallerPcSlotFromFp = 1;
|
|
static const int kParamEndSlotFromFp = 1; // One slot past last parameter.
|
|
static const int kCallerSpSlotFromFp = 2;
|
|
static const int kLastParamSlotFromEntrySp = 0;
|
|
|
|
// Entry and exit frame layout.
|
|
#if defined(TARGET_OS_MACOS) || defined(TARGET_OS_MACOS_IOS)
|
|
static const int kExitLinkSlotFromEntryFp = -27;
|
|
COMPILE_ASSERT(kAbiPreservedCpuRegCount == 6);
|
|
COMPILE_ASSERT(kAbiPreservedFpuRegCount == 4);
|
|
#else
|
|
static const int kExitLinkSlotFromEntryFp = -28;
|
|
COMPILE_ASSERT(kAbiPreservedCpuRegCount == 7);
|
|
COMPILE_ASSERT(kAbiPreservedFpuRegCount == 4);
|
|
#endif
|
|
|
|
// For FFI native -> Dart callbacks, the number of stack slots between arguments
|
|
// passed on stack and arguments saved in callback prologue.
|
|
//
|
|
// 2 = return adddress (1) + saved frame pointer (1).
|
|
//
|
|
// If NativeCallbackTrampolines::Enabled(), then
|
|
// kNativeCallbackTrampolineStackDelta must be added as well.
|
|
constexpr intptr_t kCallbackSlotsBeforeSavedArguments = 2;
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_STACK_FRAME_ARM_H_
|