mirror of
https://github.com/dart-lang/sdk
synced 2024-09-18 22:01:19 +00:00
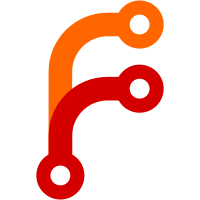
Adds support for `asset: 'asset-id'` in `FfiNative`s. Symbols in assets are resolved by 1. the provided [asset] (the bulk of this CL), 2. the native resolver set with `Dart_SetFfiNativeResolver` in `dart_api.h` (old behavior), and 3. the current process. Symbols in assets are resolved by the Dart VM through the mapping provided in the `vm:ffi:native_assets` library: ``` @pragma('vm:ffi:native-assets', { "linux_x64": { "<asset1>": [ "absolute", "<absolute-path>" ], "<asset2>": [ "relative", "<relative-path>" ], "<asset3>": [ "system", "<system-path>" ], "<asset4>": [ "process", ], "<asset5>": [ "executable", ], } }) library; ``` There are 5 asset path types. Symbols resolved in these asset types are resolved as follows. A. Absolute paths are dlopened, after which the symbol is looked up. B. Relative paths are resolved relative to `Platform.script.uri`, and dlopened, after which the symbol is looked up. C. System paths are treated as absolute paths. (But we might explicitly try to use `PATH` in the future.) D. Symbols looked up in Process assets are looked up in the current process. E. Symbols looked up in Executable assets are looked up in the current executable. The native assets mapping can be provided in three ways. X. In the invocation to `gen_kernel` with the `--native-assets` argument. This uses the gen_kernel entry point for compiling kernel. Y. By placing a `native_assets.yaml` next to the `package_config.json` used by the isolate group. This works for `dart <source file>` and `Isolate.spawnUri(<dart source file>)`. This uses the kernel_service entry point for compiling kernel. Z. By sending a String containing the native assets mapping when invoking the kernel_service directly from within the VM. Currently, only used for unit tests. TEST=tests/ffi/native_assets/asset_*_test.dart (X) TEST=tests/ffi/native_assets/infer_native_assets_yaml_*.dart (Y) TEST=runtime/vm/ffi/native_assets_test.cc (Z) The library is synthesized from yaml in pkg:vm as AST. Design doc: go/dart-native-assets Bug: https://github.com/dart-lang/sdk/issues/49803 Change-Id: I8bf7000bfcc03b362948efd3baf168838948e6b9 Cq-Include-Trybots: luci.dart.try:vm-ffi-android-debug-arm-try,vm-ffi-android-debug-arm64c-try,vm-precomp-ffi-qemu-linux-release-arm-try,vm-kernel-nnbd-mac-debug-arm64-try,vm-kernel-nnbd-mac-debug-x64-try,vm-kernel-win-debug-x64-try,vm-kernel-win-debug-ia32-try,vm-kernel-precomp-win-debug-x64c-try,vm-kernel-reload-linux-debug-x64-try,vm-kernel-reload-rollback-linux-debug-x64-try,vm-kernel-precomp-nnbd-linux-debug-x64-try,vm-kernel-precomp-win-debug-x64c-try,vm-kernel-precomp-nnbd-mac-release-arm64-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/264842 Reviewed-by: Martin Kustermann <kustermann@google.com>
24 lines
658 B
Dart
24 lines
658 B
Dart
// Copyright (c) 2023, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'dart:ffi';
|
|
import 'dart:io';
|
|
|
|
// Add an unused import to see that we're using actually the package config.
|
|
// ignore: unused_import
|
|
import 'package:meta/meta.dart';
|
|
|
|
void main() {
|
|
print('run tests');
|
|
print('Platform.packageConfig: ${Platform.packageConfig}');
|
|
|
|
final result = sumPlus42(3, 4);
|
|
print(result);
|
|
|
|
print('run done');
|
|
}
|
|
|
|
@FfiNative<Int32 Function(Int32, Int32)>('SumPlus42')
|
|
external int sumPlus42(int a, int b);
|