mirror of
https://github.com/dart-lang/sdk
synced 2024-09-15 22:41:41 +00:00
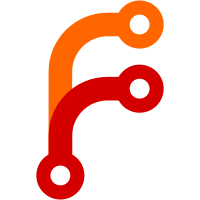
This reverts https://dart-review.googlesource.com/c/sdk/+/306674 Patchset 1 is a pure rollback of the rollback. Patchset 2 is https://dart-review.googlesource.com/c/sdk/+/306316 Patchset 4+ is the forward fix for the Fuchsia issues. The Fuchsia bug that we're fixing (or working around), is that VirtualMemory::DuplicateRX doesn't work on Fuchsia. A proper fix will require special casing it, like on MacOS. In the mean time we can avoid using this function by only allowing one page of trampolines on Fuchsia. Unfortunately, when I removed the BSS stuff from the original CL, it was necessary to duplicate even the first page, so I've had to add that stuff back just for Fuchsia. Change-Id: Id42de78ee5de126bcc83bfa4148f6efb4045f976 Bug: https://github.com/dart-lang/sdk/issues/52579 Bug: https://buganizer.corp.google.com/issues/284959841 Fixes: https://github.com/dart-lang/sdk/issues/52581 TEST=CI, especially vm-fuchsia-release-x64-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/306676 Reviewed-by: Ryan Macnak <rmacnak@google.com> Commit-Queue: Liam Appelbe <liama@google.com> Reviewed-by: Daco Harkes <dacoharkes@google.com>
65 lines
1.8 KiB
C++
65 lines
1.8 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#include "vm/allocation.h"
|
|
|
|
#include "platform/assert.h"
|
|
#include "vm/isolate.h"
|
|
#include "vm/thread.h"
|
|
#include "vm/zone.h"
|
|
|
|
namespace dart {
|
|
|
|
static void* Allocate(uword size, Zone* zone) {
|
|
ASSERT(zone != nullptr);
|
|
if (size > static_cast<uword>(kIntptrMax)) {
|
|
FATAL("ZoneAllocated object has unexpectedly large size %" Pu "", size);
|
|
}
|
|
return reinterpret_cast<void*>(zone->AllocUnsafe(size));
|
|
}
|
|
|
|
void* ZoneAllocated::operator new(uword size) {
|
|
return Allocate(size, Thread::Current()->zone());
|
|
}
|
|
|
|
void* ZoneAllocated::operator new(uword size, Zone* zone) {
|
|
return Allocate(size, zone);
|
|
}
|
|
|
|
StackResource::~StackResource() {
|
|
if (thread_ != nullptr) {
|
|
StackResource* top = thread_->top_resource();
|
|
ASSERT(top == this);
|
|
thread_->set_top_resource(previous_);
|
|
}
|
|
#if defined(DEBUG)
|
|
if (thread_ != nullptr) {
|
|
ASSERT(Thread::Current() == thread_);
|
|
}
|
|
#endif
|
|
}
|
|
|
|
void StackResource::Init(ThreadState* thread) {
|
|
// We can only have longjumps and exceptions when there is a current
|
|
// thread and isolate. If there is no current thread, we don't need to
|
|
// protect this case.
|
|
if (thread != nullptr) {
|
|
ASSERT(Thread::Current() == thread);
|
|
thread_ = thread;
|
|
previous_ = thread_->top_resource();
|
|
ASSERT((previous_ == nullptr) || (previous_->thread_ == thread));
|
|
thread_->set_top_resource(this);
|
|
}
|
|
}
|
|
|
|
void StackResource::UnwindAbove(ThreadState* thread, StackResource* new_top) {
|
|
StackResource* current_resource = thread->top_resource();
|
|
while (current_resource != new_top) {
|
|
current_resource->~StackResource();
|
|
current_resource = thread->top_resource();
|
|
}
|
|
}
|
|
|
|
} // namespace dart
|