mirror of
https://github.com/dart-lang/sdk
synced 2024-09-19 14:32:49 +00:00
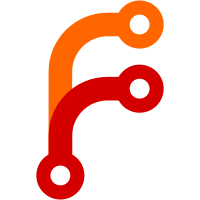
The precedence-based pattern parser can understand a unary pattern inside another unary pattern (e.g. `_ as int as num`) or a relational pattern inside a unary pattern (e.g. `> 1?`), but the specification prohibits these constructions because they're difficult to read and not very useful. This change updates the implementation to match the spec, by producing the appropriate error. The offset and length of the error cover the inner pattern, so it should be easy to construct an analysis server quick fix that inserts the necessary parentheses. Bug: https://github.com/dart-lang/sdk/issues/50035 Change-Id: I33e74d6d1f863e7162851d26fefbacd4fd17277c Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/292120 Reviewed-by: Konstantin Shcheglov <scheglov@google.com> Commit-Queue: Paul Berry <paulberry@google.com>
124 lines
2.3 KiB
Dart
124 lines
2.3 KiB
Dart
// Copyright (c) 2023, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// SharedOptions=--enable-experiment=patterns
|
|
|
|
// Test that a unary pattern or a relational pattern may appear inside a unary
|
|
// pattern as long as there are parentheses.
|
|
|
|
import 'package:expect/expect.dart';
|
|
|
|
test_cast_insideCast(x) {
|
|
switch (x) {
|
|
case (_ as int) as num:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
test_cast_insideNullAssert(x) {
|
|
switch (x) {
|
|
case (_ as int)!:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
test_cast_insideNullCheck(x) {
|
|
switch (x) {
|
|
case (_ as int?)?:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
test_nullAssert_insideCast(x) {
|
|
switch (x) {
|
|
case (_!) as num?:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
test_nullAssert_insideNullAssert(x) {
|
|
switch (x) {
|
|
case (_!)!:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
test_nullAssert_insideNullCheck(x) {
|
|
switch (x) {
|
|
case (_!)?:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
test_nullCheck_insideCast(x) {
|
|
switch (x) {
|
|
case (_?) as num?:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
test_nullCheck_insideNullAssert(x) {
|
|
switch (x) {
|
|
case (_?)!:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
test_nullCheck_insideNullCheck(x) {
|
|
switch (x) {
|
|
case (_?)?:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
test_relational_insideNullCheck_equal(x) {
|
|
switch (x) {
|
|
case (== 1)?:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
test_relational_insideNullCheck_greaterThan(x) {
|
|
switch (x) {
|
|
case (> 1)?:
|
|
break;
|
|
default:
|
|
Expect.fail('failed to match');
|
|
}
|
|
}
|
|
|
|
main() {
|
|
test_cast_insideCast(0);
|
|
test_cast_insideNullAssert(0);
|
|
test_cast_insideNullCheck(0);
|
|
test_nullAssert_insideCast(0);
|
|
test_nullAssert_insideNullAssert(0);
|
|
test_nullAssert_insideNullCheck(0);
|
|
test_nullCheck_insideCast(0);
|
|
test_nullCheck_insideNullAssert(0);
|
|
test_nullCheck_insideNullCheck(0);
|
|
test_relational_insideNullCheck_equal(1);
|
|
test_relational_insideNullCheck_greaterThan(2);
|
|
}
|