mirror of
https://github.com/dart-lang/sdk
synced 2024-09-19 21:01:50 +00:00
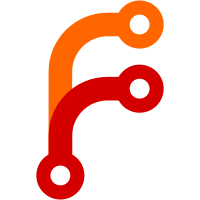
> Header files should be self-contained (compile on their own) and end in .h. Non-header files that are meant for inclusion should end in .inc and be used sparingly. > > All header files should be self-contained. Users and refactoring tools should not have to adhere to special conditions to include the header. Specifically, a header should have header guards and include all other headers it needs. This cleans up header files referring to types and functions defined in the files including those header files. This does not clean up the include guards in xyz_<arch>.h. We have currently 45 such include guards. We can clean that up by moving the #ifdefs from xyz.h to the individual xyz_<arch>.h files. A list of header files which cannot stand on itself is maintained in runtime/tools/run_clang_tidy.dart. Change-Id: I9ab1fc09056d86e8ac59a80c063a812633cf7c71 Cq-Include-Trybots: luci.dart.try:vm-ffi-android-debug-arm-try,vm-ffi-android-debug-arm64-try,app-kernel-linux-debug-x64-try,vm-kernel-linux-debug-ia32-try,vm-kernel-win-debug-x64-try,vm-kernel-win-debug-ia32-try,vm-kernel-precomp-linux-debug-x64-try,vm-dartkb-linux-release-x64-abi-try,vm-kernel-precomp-android-release-arm64-try,vm-kernel-asan-linux-release-x64-try,vm-kernel-linux-release-simarm-try,vm-kernel-linux-release-simarm64-try,vm-kernel-precomp-android-release-arm_x64-try,vm-kernel-precomp-obfuscate-linux-release-x64-try,dart-sdk-linux-try,analyzer-analysis-server-linux-try,analyzer-linux-release-try,front-end-linux-release-x64-try,vm-kernel-precomp-win-release-x64-try,vm-kernel-mac-debug-x64-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/135650 Commit-Queue: Daco Harkes <dacoharkes@google.com> Reviewed-by: Alexander Markov <alexmarkov@google.com>
36 lines
1.1 KiB
C++
36 lines
1.1 KiB
C++
// Copyright (c) 2020, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_CONSTANTS_BASE_H_
|
|
#define RUNTIME_VM_CONSTANTS_BASE_H_
|
|
|
|
namespace dart {
|
|
|
|
// Alignment strategies for how to align values.
|
|
enum AlignmentStrategy {
|
|
// Align to the size of the value.
|
|
kAlignedToValueSize,
|
|
// Align to the size of the value, but align 8 byte-sized values to 4 bytes.
|
|
// Both double and int64.
|
|
kAlignedToValueSizeBut8AlignedTo4,
|
|
// Align to the architecture size.
|
|
kAlignedToWordSize,
|
|
// Align to the architecture size, but align 8 byte-sized values to 8 bytes.
|
|
// Both double and int64.
|
|
kAlignedToWordSizeBut8AlignedTo8,
|
|
};
|
|
|
|
// Minimum size strategies for how to store values.
|
|
enum ExtensionStrategy {
|
|
// Values can have arbitrary small size with the upper bits undefined.
|
|
kNotExtended,
|
|
// Values smaller than 4 bytes are passed around zero- or signextended to
|
|
// 4 bytes.
|
|
kExtendedTo4,
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_CONSTANTS_BASE_H_
|