mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 01:30:32 +00:00
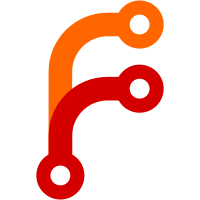
This is a reland of commit ebc96ecb83
Angular codegen emits members that are external so DDC's external
checks need to be more specific to only focus on JS interop. This
also modifies some code logic around whether a member is JS interop
to be less permissive. Before, it claimed that any member is JS
interop if it's external and the enclosing library has a @JS
annotation, but this is only true if there is no surrounding class.
Original change's description:
> [ddc] Don't emit type args on interop invocations
>
> DDC passes type args as the first args for a generic function
> invocation, but interop functions should be excluded from doing
> so, as this leads to passing type args to JS functions.
>
> Change-Id: Ia011beca8c7a0ebb3a7e6c81cd960096045c3a06
> Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/323941
> Reviewed-by: Nicholas Shahan <nshahan@google.com>
> Commit-Queue: Srujan Gaddam <srujzs@google.com>
Change-Id: Id48337ac4980d9de5de03d895d93cad3ab3a1808
Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/324542
Reviewed-by: Nicholas Shahan <nshahan@google.com>
Commit-Queue: Srujan Gaddam <srujzs@google.com>
51 lines
1.4 KiB
Dart
51 lines
1.4 KiB
Dart
// Copyright (c) 2023, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// Check that the compilers lower interop calls that use type parameters do not
|
|
// pass the type parameter.
|
|
|
|
@JS()
|
|
library type_parameter_lowering_test;
|
|
|
|
import 'package:js/js.dart';
|
|
|
|
@JS()
|
|
external void eval(String code);
|
|
|
|
@JS()
|
|
external void topLevelMethod<T extends int>(T t);
|
|
|
|
@JS()
|
|
class TypeParam<T extends int> {
|
|
external TypeParam(T t);
|
|
external static void staticMethod<U extends int>(U u);
|
|
external static void staticMethodShadow<T extends int>(T t);
|
|
external void genericMethod<U extends int>(U u);
|
|
external void genericMethodShadow<T extends int>(T t);
|
|
}
|
|
|
|
void main() {
|
|
eval('''
|
|
const checkValue = function(value) {
|
|
if (value != 0) {
|
|
throw new Error(`Expected value to be 0, but got \${value}.`);
|
|
}
|
|
}
|
|
globalThis.topLevelMethod = checkValue;
|
|
globalThis.TypeParam = function (value) {
|
|
checkValue(value);
|
|
this.genericMethod = checkValue;
|
|
this.genericMethodShadow = checkValue;
|
|
}
|
|
globalThis.TypeParam.staticMethod = checkValue;
|
|
globalThis.TypeParam.staticMethodShadow = checkValue;
|
|
''');
|
|
topLevelMethod(0);
|
|
final typeParam = TypeParam(0);
|
|
TypeParam.staticMethod(0);
|
|
TypeParam.staticMethodShadow(0);
|
|
typeParam.genericMethod(0);
|
|
typeParam.genericMethodShadow(0);
|
|
}
|