mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
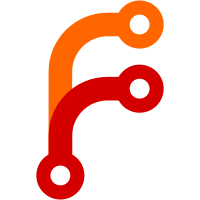
https://github.com/dart-lang/sdk/issues/54004 In order to reach parity with js_util, we expose operators through an extension and do some partial renames in order to make the member names sound better e.g. `equals` instead of `equal`. We also expose the following from js_util: - NullRejectionException We don't expose `isJavaScriptArray` and `isJavaScriptSimpleObject` as they can expressed through other forms of interop. There was an esoteric bug where we needed these members for Firefox in pkg:test, but the package no longer uses these members, so to avoid increasing the API space too much, we do not export them. For the same reason, we also don't expose `objectGetPrototypeOf`, `objectPrototype`, `objectKeys`. We don't expose `allowInteropCaptureThis` as it will take some work to handle this in dart2wasm. That work is tracked in https://github.com/dart-lang/sdk/issues/54381. Lastly, `instanceof` and `instanceOfString` is moved to be on `JSAny?`, as this operator is useful to avoid needing to downcast to `JSObject` e.g. `any.instanceOfString('Window')` instead of `any.typeofEquals('object') && (any as JSObject).instanceOfString('Window')`. Extensions are reorganized and renamed to handle these changes. CoreLibraryReviewExempt: Backend-specific library. Change-Id: Ib1a7fabc3fa985ef6638620becccd27eeca68c25 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/341140 Reviewed-by: Sigmund Cherem <sigmund@google.com>
145 lines
8.5 KiB
Dart
145 lines
8.5 KiB
Dart
// Copyright (c) 2023, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
@JS()
|
|
library operator_test;
|
|
|
|
import 'package:js/js.dart';
|
|
|
|
@JS()
|
|
class JSClass {
|
|
// https://dart.dev/guides/language/language-tour#_operators for the list of
|
|
// operators allowed by the language.
|
|
@JS('rename')
|
|
external void operator <(_);
|
|
// ^
|
|
// [web] JS interop operator methods cannot be renamed using the '@JS' annotation.
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator >(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator <=(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator >=(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator -(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator +(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator /(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator ~/(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator *(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator %(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator |(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator ^(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator &(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator <<(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator >>(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator >>>(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator [](_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator []=(_, __);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator ~();
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external bool operator ==(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
}
|
|
|
|
@JS()
|
|
@anonymous
|
|
class AnonymousClass {
|
|
@JS('rename')
|
|
external void operator <(_);
|
|
// ^
|
|
// [web] JS interop operator methods cannot be renamed using the '@JS' annotation.
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator >(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator <=(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator >=(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator -(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator +(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator /(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator ~/(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator *(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator %(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator |(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator ^(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator &(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator <<(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator >>(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator >>>(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator [](_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator []=(_, __);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external void operator ~();
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
external bool operator ==(_);
|
|
// ^
|
|
// [web] JS interop types do not support overloading external operator methods, with the exception of '[]' and '[]=' using static interop.
|
|
}
|
|
|
|
void main() {}
|