mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
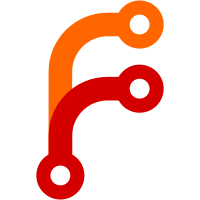
Split out async, jsify, and html tests. Also split JS$ prefix test and skipped by design for ddc which doesn't support the prefix. Change-Id: I85c673d791731bbae3ff4015a7e134d9180170de Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/185281 Commit-Queue: Riley Porter <rileyporter@google.com> Reviewed-by: Sigmund Cherem <sigmund@google.com> Reviewed-by: Srujan Gaddam <srujzs@google.com>
90 lines
2.5 KiB
Dart
90 lines
2.5 KiB
Dart
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// Tests the functionality of js_util with HTML objects.
|
|
|
|
@JS()
|
|
library js_util_test;
|
|
|
|
import 'dart:html';
|
|
|
|
import 'package:js/js.dart';
|
|
import 'package:js/js_util.dart' as js_util;
|
|
import 'package:expect/minitest.dart';
|
|
|
|
@JS()
|
|
external void eval(String code);
|
|
|
|
@JS('Node')
|
|
external get JSNodeType;
|
|
|
|
@JS('Element')
|
|
external get JSElementType;
|
|
|
|
@JS('Text')
|
|
external get JSTextType;
|
|
|
|
@JS('HTMLCanvasElement')
|
|
external get JSHtmlCanvasElementType;
|
|
|
|
@JS()
|
|
class Foo {
|
|
external Foo();
|
|
}
|
|
|
|
main() {
|
|
eval(r""" function Foo() {} """);
|
|
|
|
test('hasProperty', () {
|
|
var textElement = new Text('foo');
|
|
expect(js_util.hasProperty(textElement, 'data'), isTrue);
|
|
});
|
|
|
|
test('getProperty', () {
|
|
var textElement = new Text('foo');
|
|
expect(js_util.getProperty(textElement, 'data'), equals('foo'));
|
|
});
|
|
|
|
test('setProperty', () {
|
|
var textElement = new Text('foo');
|
|
js_util.setProperty(textElement, 'data', 'bar');
|
|
expect(textElement.text, equals('bar'));
|
|
});
|
|
|
|
test('callMethod', () {
|
|
var canvas = new CanvasElement();
|
|
expect(
|
|
identical(canvas.getContext('2d'),
|
|
js_util.callMethod(canvas, 'getContext', ['2d'])),
|
|
isTrue);
|
|
});
|
|
|
|
test('instanceof', () {
|
|
var canvas = new Element.tag('canvas');
|
|
expect(js_util.instanceof(canvas, JSNodeType), isTrue);
|
|
expect(js_util.instanceof(canvas, JSTextType), isFalse);
|
|
expect(js_util.instanceof(canvas, JSElementType), isTrue);
|
|
expect(js_util.instanceof(canvas, JSHtmlCanvasElementType), isTrue);
|
|
var div = new Element.tag('div');
|
|
expect(js_util.instanceof(div, JSNodeType), isTrue);
|
|
expect(js_util.instanceof(div, JSTextType), isFalse);
|
|
expect(js_util.instanceof(div, JSElementType), isTrue);
|
|
expect(js_util.instanceof(div, JSHtmlCanvasElementType), isFalse);
|
|
|
|
var text = new Text('foo');
|
|
expect(js_util.instanceof(text, JSNodeType), isTrue);
|
|
expect(js_util.instanceof(text, JSTextType), isTrue);
|
|
expect(js_util.instanceof(text, JSElementType), isFalse);
|
|
|
|
var f = new Foo();
|
|
expect(js_util.instanceof(f, JSNodeType), isFalse);
|
|
});
|
|
|
|
test('callConstructor', () {
|
|
var textNode = js_util.callConstructor(JSTextType, ['foo']);
|
|
expect(js_util.instanceof(textNode, JSTextType), isTrue);
|
|
expect(textNode is Text, isTrue);
|
|
expect(textNode.text, equals('foo'));
|
|
});
|
|
}
|