mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 01:30:32 +00:00
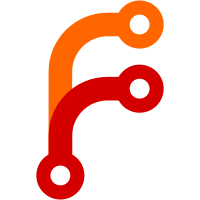
These tests had several issues. The fixes are aimed to reduce flakiness and remove invalid configurations where tests are not providing any value. Some of the issues include: * some tests interferered with each other causing a 18% flakiness rate in chrome: they used a common file system that ended up being shared in a single browser instance. This is why some tests failed when they find a file exists before it was created [1]. * some tests were not properly using async_helper/async_minitest, so they may complete before they finish executing * DDC no longer supports these APIs due to https://github.com/dart-lang/sdk/issues/53864. We don't intend to fix those issues, so there is no purpose in running these tests in DDC at this time. [1]: https://logs.chromium.org/logs/dart/buildbucket/cr-buildbucket/8766313714239949697/+/u/test_results/ignored_flaky_test_failure_logs Change-Id: I1dbb28eba2d962000a4d425563dbc1eb6b55dbed Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/332266 Commit-Queue: Sigmund Cherem <sigmund@google.com> Reviewed-by: Srujan Gaddam <srujzs@google.com>
71 lines
2.3 KiB
Dart
71 lines
2.3 KiB
Dart
// Copyright (c) 2020, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'dart:async';
|
|
import 'dart:html';
|
|
|
|
import 'package:async_helper/async_minitest.dart';
|
|
|
|
class FileAndDir {
|
|
FileEntry file;
|
|
DirectoryEntry dir;
|
|
FileAndDir(this.file, this.dir);
|
|
}
|
|
|
|
main() {
|
|
if (!FileSystem.supported) return;
|
|
// Prepend this file name to prevent collisions among tests runnning on the
|
|
// same browser.
|
|
const prefix = 'fileapi_entry_';
|
|
|
|
// Do the boilerplate to get several files and directories created to then
|
|
// test the functions that use those items.
|
|
Future doDirSetup(FileSystem fs, String testName) async {
|
|
var file =
|
|
await fs.root!.createFile('${prefix}file_$testName') as FileEntry;
|
|
var dir = await fs.root!.createDirectory('${prefix}dir_$testName')
|
|
as DirectoryEntry;
|
|
return new Future.value(new FileAndDir(file, dir));
|
|
}
|
|
|
|
test('copy_move', () async {
|
|
final fs = await window.requestFileSystem(100);
|
|
var fileAndDir = await doDirSetup(fs, 'copyTo');
|
|
var entry =
|
|
await fileAndDir.file.copyTo(fileAndDir.dir, name: 'copiedFile');
|
|
expect(entry.isFile, true, reason: "Expected File");
|
|
expect(entry.name, 'copiedFile');
|
|
|
|
// getParent
|
|
fileAndDir = await doDirSetup(fs, 'getParent');
|
|
entry = await fileAndDir.file.getParent();
|
|
expect(entry.name, '');
|
|
expect(entry.isDirectory, true, reason: "Expected Directory");
|
|
|
|
// moveTo
|
|
fileAndDir = await doDirSetup(fs, 'moveTo');
|
|
entry = await fileAndDir.file.moveTo(fileAndDir.dir, name: 'movedFile');
|
|
expect(entry.name, 'movedFile');
|
|
expect(entry.fullPath, '/${prefix}dir_moveTo/movedFile');
|
|
|
|
try {
|
|
entry = await fs.root!.getFile('${prefix}file4');
|
|
fail("File ${prefix}file4 should not exist.");
|
|
} on DomException catch (error) {
|
|
expect(DomException.NOT_FOUND, error.name);
|
|
}
|
|
|
|
// remove
|
|
fileAndDir = await doDirSetup(fs, 'remove');
|
|
expect('${prefix}file_remove', fileAndDir.file.name);
|
|
await fileAndDir.file.remove();
|
|
try {
|
|
await fileAndDir.dir.getFile(fileAndDir.file.name);
|
|
fail("file not removed");
|
|
} on DomException catch (error) {
|
|
expect(DomException.NOT_FOUND, error.name);
|
|
}
|
|
});
|
|
}
|