mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
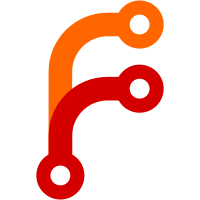
This reverts commitf0a8b63402
. Reason for revert: The Flutter SDK issues have been resolved - see https://github.com/flutter/flutter/issues/80969 and https://github.com/flutter/flutter/pull/81014 Original change's description: > Revert "Make throwing Zone.uncaughtError handlers propagate the error to their parent zone." > > This reverts commit88a351f3d2
. > > This broke the Dart SDK -> Flutter Engine roller. Flutter issue at https://github.com/flutter/flutter/issues/80969 > > Change-Id: Idaf255a730c7b6054e6cd929b6770dbe66860151 > Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/196561 > Reviewed-by: Zach Anderson <zra@google.com> > Commit-Queue: Zach Anderson <zra@google.com> # Not skipping CQ checks because this is a reland. Change-Id: Icd98c550c63160f35cc5da40af7ca6bf2cbf180e Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/196621 Reviewed-by: Dan Field <dnfield@google.com> Reviewed-by: Siva Annamalai <asiva@google.com> Commit-Queue: Dan Field <dnfield@google.com>
76 lines
2.5 KiB
Dart
76 lines
2.5 KiB
Dart
// Copyright (c) 2021, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'package:expect/expect.dart';
|
|
import 'package:async_helper/async_helper.dart';
|
|
import 'dart:async';
|
|
|
|
void main() async {
|
|
asyncStart();
|
|
await testThrowSame();
|
|
await testThrowOther();
|
|
asyncEnd();
|
|
}
|
|
|
|
Future<void> testThrowSame() async {
|
|
asyncStart();
|
|
var object1 = Object();
|
|
var stack1 = StackTrace.current;
|
|
var outerZone = Zone.current;
|
|
var firstZone = Zone.current.fork(specification: onError((error, stack) {
|
|
// Uncaught error handlers run in the parent zone.
|
|
Expect.identical(outerZone, Zone.current);
|
|
Expect.identical(object1, error);
|
|
Expect.identical(stack1, stack); // Get same stack trace.
|
|
asyncEnd();
|
|
}));
|
|
firstZone.run(() async {
|
|
Expect.identical(firstZone, Zone.current);
|
|
var secondZone = Zone.current.fork(specification: onError((error, stack) {
|
|
// Uncaught error handlers run in the parent zone.
|
|
Expect.identical(firstZone, Zone.current);
|
|
Expect.identical(object1, error);
|
|
Expect.identical(stack1, stack);
|
|
throw error; // Throw same object
|
|
}));
|
|
secondZone.run(() async {
|
|
Expect.identical(secondZone, Zone.current);
|
|
Future.error(object1, stack1); // Unhandled async error.
|
|
await Future(() {});
|
|
});
|
|
});
|
|
}
|
|
|
|
Future<void> testThrowOther() async {
|
|
asyncStart();
|
|
var object1 = Object();
|
|
var object2 = Object();
|
|
var stack1 = StackTrace.current;
|
|
var outerZone = Zone.current;
|
|
var firstZone = Zone.current.fork(specification: onError((error, stack) {
|
|
Expect.identical(outerZone, Zone.current);
|
|
Expect.identical(object2, error);
|
|
Expect.notIdentical(stack1, stack); // Get different stack trace.
|
|
asyncEnd();
|
|
}));
|
|
firstZone.run(() async {
|
|
Expect.identical(firstZone, Zone.current);
|
|
var secondZone = Zone.current.fork(specification: onError((error, stack) {
|
|
Expect.identical(firstZone, Zone.current);
|
|
Expect.identical(object1, error);
|
|
Expect.identical(stack1, stack);
|
|
throw object2; // Throw different object
|
|
}));
|
|
secondZone.run(() async {
|
|
Expect.identical(secondZone, Zone.current);
|
|
Future.error(object1, stack1); // Unhandled async error.
|
|
await Future(() {});
|
|
});
|
|
});
|
|
}
|
|
|
|
ZoneSpecification onError(void Function(Object, StackTrace) handler) {
|
|
return ZoneSpecification(
|
|
handleUncaughtError: (s, p, z, e, st) => handler(e, st));
|
|
}
|