mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
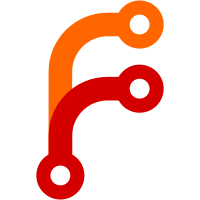
Closes #40520 Closes #40948 Closes #40425 Change-Id: I0aa3cfa51b410c90dd0bea963846eeb6b2e73efb Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/140540 Commit-Queue: Johnni Winther <johnniwinther@google.com> Reviewed-by: Dmitry Stefantsov <dmitryas@google.com>
94 lines
2.4 KiB
Dart
94 lines
2.4 KiB
Dart
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'package:async_helper/async_helper.dart';
|
|
import "package:expect/expect.dart";
|
|
import 'dart:async';
|
|
|
|
Future testOneScheduleMicrotask() {
|
|
var completer = new Completer();
|
|
Timer.run(() {
|
|
scheduleMicrotask(completer.complete);
|
|
});
|
|
return completer.future;
|
|
}
|
|
|
|
Future testMultipleScheduleMicrotask() {
|
|
var completer = new Completer();
|
|
Timer.run(() {
|
|
const TOTAL = 10;
|
|
int done = 0;
|
|
for (int i = 0; i < TOTAL; i++) {
|
|
scheduleMicrotask(() {
|
|
done++;
|
|
if (done == TOTAL) completer.complete();
|
|
;
|
|
});
|
|
}
|
|
});
|
|
return completer.future;
|
|
}
|
|
|
|
Future testScheduleMicrotaskThenTimer() {
|
|
var completer = new Completer();
|
|
Timer.run(() {
|
|
bool scheduleMicrotaskDone = false;
|
|
scheduleMicrotask(() {
|
|
Expect.isFalse(scheduleMicrotaskDone);
|
|
scheduleMicrotaskDone = true;
|
|
});
|
|
Timer.run(() {
|
|
Expect.isTrue(scheduleMicrotaskDone);
|
|
completer.complete();
|
|
});
|
|
});
|
|
return completer.future;
|
|
}
|
|
|
|
Future testTimerThenScheduleMicrotask() {
|
|
var completer = new Completer();
|
|
Timer.run(() {
|
|
bool scheduleMicrotaskDone = false;
|
|
Timer.run(() {
|
|
Expect.isTrue(scheduleMicrotaskDone);
|
|
completer.complete();
|
|
});
|
|
scheduleMicrotask(() {
|
|
Expect.isFalse(scheduleMicrotaskDone);
|
|
scheduleMicrotaskDone = true;
|
|
});
|
|
});
|
|
return completer.future;
|
|
}
|
|
|
|
Future testTimerThenScheduleMicrotaskChain() {
|
|
var completer = new Completer();
|
|
Timer.run(() {
|
|
const TOTAL = 10;
|
|
int scheduleMicrotaskDone = 0;
|
|
Timer.run(() {
|
|
Expect.equals(TOTAL, scheduleMicrotaskDone);
|
|
completer.complete();
|
|
});
|
|
void scheduleMicrotaskCallback() {
|
|
scheduleMicrotaskDone++;
|
|
if (scheduleMicrotaskDone != TOTAL) {
|
|
scheduleMicrotask(scheduleMicrotaskCallback);
|
|
}
|
|
}
|
|
|
|
scheduleMicrotask(scheduleMicrotaskCallback);
|
|
});
|
|
return completer.future;
|
|
}
|
|
|
|
main() {
|
|
asyncStart();
|
|
testOneScheduleMicrotask()
|
|
.then((_) => testMultipleScheduleMicrotask())
|
|
.then((_) => testScheduleMicrotaskThenTimer())
|
|
.then((_) => testTimerThenScheduleMicrotask())
|
|
.then((_) => testTimerThenScheduleMicrotaskChain())
|
|
.then((_) => asyncEnd());
|
|
}
|