mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 08:24:39 +00:00
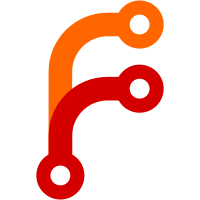
Don't emit dead code branches that are guarded by boolean literals. Add a single level of simplification for !true -> false, and !false -> true. While this might not be very common in code, it does allow for a useful pattern in the runtime libraries. Branches can be included into the compiled code or not based on a compile time flag. Change-Id: Ib90e1e951cea3ef8c75b944635776b292759594a Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/243363 Commit-Queue: Nicholas Shahan <nshahan@google.com> Reviewed-by: Anna Gringauze <annagrin@google.com>
55 lines
1.7 KiB
Dart
55 lines
1.7 KiB
Dart
// Copyright (c) 2019, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'dart:_foreign_helper' show JS;
|
|
|
|
import 'package:expect/expect.dart';
|
|
|
|
void main() async {
|
|
var count = 0;
|
|
if (JS<bool>('!', 'false')) {
|
|
// Should be eliminated from the output based on the condition above.
|
|
JS('', 'syntax error here!');
|
|
}
|
|
count++;
|
|
if (JS<bool>('!', 'true')) {
|
|
count++;
|
|
} else {
|
|
// Should be eliminated from the output based on the condition above.
|
|
JS('', 'syntax error here!');
|
|
}
|
|
if (JS<bool>('!', 'false')) {
|
|
// Should be eliminated from the output based on the condition above.
|
|
JS('', 'syntax error here!');
|
|
} else {
|
|
count++;
|
|
}
|
|
if (!JS<bool>('!', 'true')) {
|
|
// Should be eliminated from the output based on the condition above.
|
|
JS('', 'syntax error here!');
|
|
}
|
|
count++;
|
|
if (!JS<bool>('!', 'false')) {
|
|
count++;
|
|
} else {
|
|
// Should be eliminated from the output based on the condition above.
|
|
JS('', 'syntax error here!');
|
|
}
|
|
if (!JS<bool>('!', 'true')) {
|
|
// Should be eliminated from the output based on the condition above.
|
|
JS('', 'syntax error here!');
|
|
} else {
|
|
count++;
|
|
}
|
|
|
|
JS<bool>('!', 'true') ? count++ : JS('', 'syntax error here!');
|
|
JS<bool>('!', 'false') ? JS('', 'syntax error here!') : count++;
|
|
!JS<bool>('!', 'true') ? JS('', 'syntax error here!') : count++;
|
|
!JS<bool>('!', 'false') ? count++ : JS('', 'syntax error here!');
|
|
|
|
// All expected branches are evaluated, and none of the syntax errors where
|
|
// compiled at all.
|
|
Expect.equals(10, count);
|
|
}
|