mirror of
https://github.com/dart-lang/sdk
synced 2024-09-19 20:41:45 +00:00
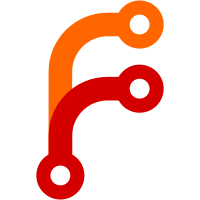
This change makes CTX available by not caching the current context while in Dart code. Instead the current context is held in a local variable (:saved_current_context_var) and is passed as argument in CTX at calls. This also simplifies a lot of code in the debugger: As a result, Isolate::top_context is not needed anymore since the current context can always be extracted from a Dart frame. R=vegorov@google.com Review URL: https://codereview.chromium.org//678763004 git-svn-id: https://dart.googlecode.com/svn/branches/bleeding_edge/dart@41422 260f80e4-7a28-3924-810f-c04153c831b5
50 lines
1.8 KiB
C++
50 lines
1.8 KiB
C++
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef VM_STACK_FRAME_IA32_H_
|
|
#define VM_STACK_FRAME_IA32_H_
|
|
|
|
namespace dart {
|
|
|
|
/* IA32 Dart Frame Layout
|
|
|
|
| | <- TOS
|
|
Callee frame | ... |
|
|
| saved EBP | (EBP of current frame)
|
|
| saved PC | (PC of current frame)
|
|
+--------------------+
|
|
Current frame | ... T| <- ESP of current frame
|
|
| first local T|
|
|
| PC marker | (current frame's code entry + offset)
|
|
| caller's EBP | <- EBP of current frame
|
|
| caller's ret addr | (PC of caller frame)
|
|
+--------------------+
|
|
Caller frame | last parameter | <- ESP of caller frame
|
|
| ... |
|
|
|
|
T against a slot indicates it needs to be traversed during GC.
|
|
*/
|
|
|
|
static const int kDartFrameFixedSize = 3; // PC marker, EBP, PC.
|
|
static const int kSavedPcSlotFromSp = -1;
|
|
|
|
static const int kFirstObjectSlotFromFp = -2; // Used by GC to traverse stack.
|
|
|
|
static const int kFirstLocalSlotFromFp = -2;
|
|
static const int kPcMarkerSlotFromFp = -1;
|
|
static const int kSavedCallerFpSlotFromFp = 0;
|
|
static const int kSavedCallerPcSlotFromFp = 1;
|
|
static const int kParamEndSlotFromFp = 1; // One slot past last parameter.
|
|
static const int kCallerSpSlotFromFp = 2;
|
|
|
|
// No pool pointer on IA32 (indicated by aliasing saved fp).
|
|
static const int kSavedCallerPpSlotFromFp = kSavedCallerFpSlotFromFp;
|
|
|
|
// Entry and exit frame layout.
|
|
static const int kExitLinkSlotFromEntryFp = -5;
|
|
|
|
} // namespace dart
|
|
|
|
#endif // VM_STACK_FRAME_IA32_H_
|