mirror of
https://github.com/dart-lang/sdk
synced 2024-10-06 16:19:07 +00:00
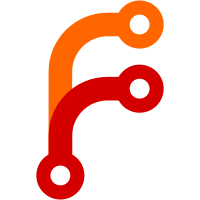
In addition, this removes support for seeding the VM isolate snapshot with Instructions and referencing those Instructions in the isolate snapshot. This was leftover from an earlier experiment to share Instructions between a Core-JIT snapshot and App-JIT snapshots. Removing this reclaims the sign bit on Instruction offsets. Add missing cases to TypeTestingStubFinder::StubNameFromAddresss. Change-Id: Ie87216b4e284db1dc3eddb12f38ddbe8a841d312 Reviewed-on: https://dart-review.googlesource.com/50620 Commit-Queue: Ryan Macnak <rmacnak@google.com> Reviewed-by: Alexander Markov <alexmarkov@google.com>
31 lines
1,008 B
C++
31 lines
1,008 B
C++
// Copyright (c) 2018, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_HASH_H_
|
|
#define RUNTIME_VM_HASH_H_
|
|
|
|
namespace dart {
|
|
|
|
static uint32_t CombineHashes(uint32_t hash, uint32_t other_hash) {
|
|
hash += other_hash;
|
|
hash += hash << 10;
|
|
hash ^= hash >> 6; // Logical shift, unsigned hash.
|
|
return hash;
|
|
}
|
|
|
|
static uint32_t FinalizeHash(uint32_t hash, intptr_t hashbits) {
|
|
hash += hash << 3;
|
|
hash ^= hash >> 11; // Logical shift, unsigned hash.
|
|
hash += hash << 15;
|
|
// FinalizeHash gets called with values for hashbits that are bigger than 31
|
|
// (like kBitsPerWord - 1). Therefore we are careful to use a type
|
|
// (uintptr_t) big enough to avoid undefined behavior with the left shift.
|
|
hash &= (static_cast<uintptr_t>(1) << hashbits) - 1;
|
|
return (hash == 0) ? 1 : hash;
|
|
}
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_HASH_H_
|