mirror of
https://github.com/dart-lang/sdk
synced 2024-11-05 18:22:09 +00:00
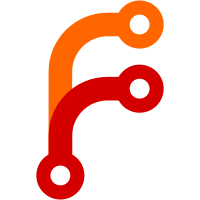
Fixes #33498 Bug: http://dartbug.com/33498 Change-Id: I4a62a830cc8993748da816967753a8ce9a025d46 Reviewed-on: https://dart-review.googlesource.com/61500 Commit-Queue: Dmitry Stefantsov <dmitryas@google.com> Reviewed-by: Lasse R.H. Nielsen <lrn@google.com>
47 lines
1.1 KiB
Dart
47 lines
1.1 KiB
Dart
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// Test for unresolved super[].
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
class A {
|
|
var indexField = new List(2);
|
|
operator []=(index, value) {
|
|
indexField[index] = value;
|
|
}
|
|
|
|
operator [](index);
|
|
|
|
noSuchMethod(Invocation im) {
|
|
if (im.memberName == const Symbol('[]')) {
|
|
Expect.equals(1, im.positionalArguments.length);
|
|
return indexField[im.positionalArguments[0]];
|
|
} else {
|
|
Expect.fail('Should not reach here');
|
|
}
|
|
}
|
|
}
|
|
|
|
class B extends A {
|
|
test() {
|
|
Expect.equals(42, super[0] = 42);
|
|
Expect.equals(42, super[0]);
|
|
Expect.equals(43, super[0] += 1);
|
|
Expect.equals(43, super[0]);
|
|
Expect.equals(43, super[0]++);
|
|
Expect.equals(44, super[0]);
|
|
|
|
Expect.equals(2, super[0] = 2);
|
|
Expect.equals(2, super[0]);
|
|
Expect.equals(3, super[0] += 1);
|
|
Expect.equals(3, super[0]);
|
|
Expect.equals(3, super[0]++);
|
|
Expect.equals(4, super[0]);
|
|
}
|
|
}
|
|
|
|
main() {
|
|
new B().test();
|
|
}
|