mirror of
https://github.com/dart-lang/sdk
synced 2024-11-05 18:22:09 +00:00
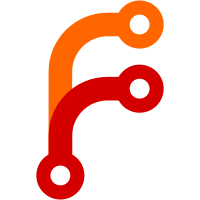
These tests break on all backends. It looks like the original intent was to run to completion. Change-Id: I895638c522285f280cbb608c82a8a496bfd11d72 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/98302 Commit-Queue: Vijay Menon <vsm@google.com> Reviewed-by: Bob Nystrom <rnystrom@google.com> Reviewed-by: Lasse R.H. Nielsen <lrn@google.com>
51 lines
1.5 KiB
Dart
51 lines
1.5 KiB
Dart
// Copyright (c) 2011, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
class ConstListTest {
|
|
static testConstructors() {
|
|
List fixedList = new List(4);
|
|
List fixedList2 = new List(4);
|
|
List growableList = new List();
|
|
List growableList2 = new List();
|
|
for (int i = 0; i < 4; i++) {
|
|
fixedList[i] = i;
|
|
fixedList2[i] = i;
|
|
growableList.add(i);
|
|
growableList2.add(i);
|
|
}
|
|
Expect.equals(growableList, growableList);
|
|
Expect.notEquals(growableList, growableList2);
|
|
Expect.equals(fixedList, fixedList);
|
|
Expect.notEquals(fixedList, fixedList2);
|
|
Expect.notEquals(fixedList, growableList);
|
|
growableList.add(4);
|
|
Expect.notEquals(fixedList, growableList);
|
|
Expect.equals(4, growableList.removeLast());
|
|
Expect.notEquals(fixedList, growableList);
|
|
fixedList[3] = 0;
|
|
Expect.notEquals(fixedList, growableList);
|
|
}
|
|
|
|
static testLiterals() {
|
|
dynamic a = [1, 2, 3.1];
|
|
dynamic b = [1, 2, 3.1];
|
|
Expect.notEquals(a, b);
|
|
a = const [1, 2, 3.1];
|
|
b = const [1, 2, 3.1];
|
|
Expect.identical(a, b);
|
|
a = const <num>[1, 2, 3.1];
|
|
b = const [1, 2, 3.1];
|
|
Expect.identical(a, b);
|
|
a = const <dynamic>[1, 2, 3.1];
|
|
b = const [1, 2, 3.1];
|
|
Expect.notEquals(a, b);
|
|
}
|
|
}
|
|
|
|
main() {
|
|
ConstListTest.testConstructors();
|
|
ConstListTest.testLiterals();
|
|
}
|