mirror of
https://github.com/dart-lang/sdk
synced 2024-09-06 00:59:09 +00:00
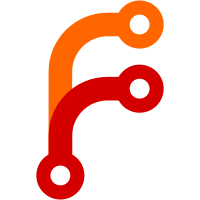
The "@compile-error" comment is an old not-great way of defining static error tests. See: https://github.com/dart-lang/sdk/issues/45634 Change-Id: I42d3712f2f75f66e7ecae19f740de16f6025b41d Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/296120 Commit-Queue: Bob Nystrom <rnystrom@google.com> Reviewed-by: Leaf Petersen <leafp@google.com>
57 lines
1.7 KiB
Dart
57 lines
1.7 KiB
Dart
// Copyright (c) 2011, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
/**
|
|
* Verify static compilation errors on strings and lists.
|
|
*/
|
|
class CoreStaticTypesTest {
|
|
static testMain() {
|
|
testStringOperators();
|
|
testStringMethods();
|
|
testListOperators();
|
|
}
|
|
|
|
static testStringOperators() {
|
|
var q = "abcdef";
|
|
q['hello'];
|
|
//^^^^^^^
|
|
// [analyzer] COMPILE_TIME_ERROR.ARGUMENT_TYPE_NOT_ASSIGNABLE
|
|
// [cfe] A value of type 'String' can't be assigned to a variable of type 'int'.
|
|
q[0] = 'x';
|
|
// ^^^
|
|
// [analyzer] COMPILE_TIME_ERROR.UNDEFINED_OPERATOR
|
|
// [cfe] The operator '[]=' isn't defined for the class 'String'.
|
|
}
|
|
|
|
static testStringMethods() {
|
|
var s = "abcdef";
|
|
s.startsWith(1);
|
|
// ^
|
|
// [analyzer] COMPILE_TIME_ERROR.ARGUMENT_TYPE_NOT_ASSIGNABLE
|
|
// [cfe] The argument type 'int' can't be assigned to the parameter type 'Pattern'.
|
|
s.endsWith(1);
|
|
// ^
|
|
// [analyzer] COMPILE_TIME_ERROR.ARGUMENT_TYPE_NOT_ASSIGNABLE
|
|
// [cfe] The argument type 'int' can't be assigned to the parameter type 'String'.
|
|
}
|
|
|
|
static testListOperators() {
|
|
var a = [1, 2, 3, 4];
|
|
a['0'];
|
|
//^^^
|
|
// [analyzer] COMPILE_TIME_ERROR.ARGUMENT_TYPE_NOT_ASSIGNABLE
|
|
// [cfe] A value of type 'String' can't be assigned to a variable of type 'int'.
|
|
a['0'] = 99;
|
|
//^^^
|
|
// [analyzer] COMPILE_TIME_ERROR.ARGUMENT_TYPE_NOT_ASSIGNABLE
|
|
// [cfe] A value of type 'String' can't be assigned to a variable of type 'int'.
|
|
}
|
|
}
|
|
|
|
main() {
|
|
CoreStaticTypesTest.testMain();
|
|
}
|