mirror of
https://github.com/dart-lang/sdk
synced 2024-10-05 04:27:25 +00:00
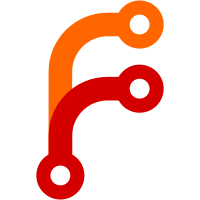
This CL implements the core flow analysis infrastructure for tracking reasons why an expression was not promoted. It supports the following reasons: - Expression was a property access - Expression has been written to since it was promoted I expect to add support for other non-promotion reasons in the future, for example: - `this` cannot be promoted - Expression has been write captured - Expression was a reference to a static field or top level variable These non-promotion reasons are plumbed through to the CFE and analyzer for the purpose of making errors easier for the user to understand. For example, given the following code: class C { int? i; f() { if (i == null) return; print(i.isEven); } } The front end now prints: ../../tmp/test.dart:5:13: Error: Property 'isEven' cannot be accessed on 'int?' because it is potentially null. Try accessing using ?. instead. print(i.isEven); ^^^^^^ Context: 'i' refers to a property so it could not be promoted. Much work still needs to be done to round out this feature, for example: - Currently the analyzer only shows the new "why not promoted" messages when the "--verbose" flag is specified; this means the feature is unlikely to be noticed by users. - Currently the analyzer doesn't show a "why not promoted" message when the non-promotion reason is that the expression is a property access. - We need one or more web pages explaining non-promotion reasons in more detail so that the error messages can contain pointers to them. - The analyzer and front end currently only show non-promotion reasons for expressions of the form `x.y` where `x` fails to be promoted to non-nullable. There are many other scenarios that should be handled. Change-Id: I0a12df74d0fc6274dfb3cb555abea81a75884231 Bug: https://github.com/dart-lang/sdk/issues/38773 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/181741 Commit-Queue: Paul Berry <paulberry@google.com> Reviewed-by: Konstantin Shcheglov <scheglov@google.com>
22 lines
903 B
Dart
22 lines
903 B
Dart
// Copyright (c) 2020, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'package:_fe_analyzer_shared/src/testing/id_testing.dart' as id;
|
|
|
|
main() async {
|
|
await id.updateAllTests(idTests);
|
|
}
|
|
|
|
const List<String> idTests = <String>[
|
|
'pkg/analyzer/test/id_tests/assigned_variables_test.dart',
|
|
'pkg/analyzer/test/id_tests/constant_test.dart',
|
|
'pkg/analyzer/test/id_tests/definite_assignment_test.dart',
|
|
'pkg/analyzer/test/id_tests/definite_unassignment_test.dart',
|
|
'pkg/analyzer/test/id_tests/inheritance_test.dart',
|
|
'pkg/analyzer/test/id_tests/nullability_test.dart',
|
|
'pkg/analyzer/test/id_tests/reachability_test.dart',
|
|
'pkg/analyzer/test/id_tests/type_promotion_test.dart',
|
|
'pkg/analyzer/test/id_tests/why_not_promoted_test.dart',
|
|
];
|