mirror of
https://github.com/dart-lang/sdk
synced 2024-10-05 05:17:31 +00:00
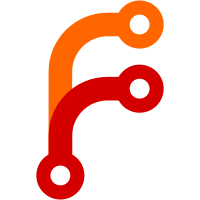
This is a reland of 5909fd111d
Does a large refactoring on the V8 snapshot profile writer
to clean things up, add more debugging support, and to fix
the problems that surfaced during the original landing.
Other changes:
Changes Serializer::CreateArtificialNodeIfNeeded() to create
artificial nodes for Code objects and immutable arrays.
Fixes CodeSerializationCluster::Trace() to only push needed parts of
discarded code objects, instead of tracing them like full code objects.
Adds test cases to v8_snapshot_profile_writer_test that exercise
the following situations (both separately and together):
* Non-symbolic stack traces are enabled and code and function objects
are dropped when not needed at runtime.
* Creation of the dispatch table is disabled.
TEST=vm/dart{,_2}/v8_snapshot_profile_writer_test
Original change's description:
> [vm] Fix V8 snapshot profile handling of the dispatch table.
>
> Fixes https://github.com/dart-lang/sdk/issues/45702.
>
> TEST=Tests listed in the issue above.
>
> Cq-Include-Trybots: luci.dart.try:vm-kernel-precomp-linux-debug-x64-try
> Change-Id: Ibf5e3ccf3828c01f9dda47de360314dabe8cb8a9
> Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/195272
> Reviewed-by: Daco Harkes <dacoharkes@google.com>
> Commit-Queue: Tess Strickland <sstrickl@google.com>
Change-Id: I8e7030267fe190079a8f68d00fe20bf7170e5719
Cq-Include-Trybots: luci.dart.try:vm-kernel-precomp-linux-debug-x64-try,vm-kernel-precomp-linux-product-x64-try,vm-kernel-precomp-mac-release-simarm64-try,vm-kernel-precomp-linux-debug-x64c-try,vm-kernel-precomp-obfuscate-linux-release-x64-try
Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/195513
Reviewed-by: Daco Harkes <dacoharkes@google.com>
Commit-Queue: Tess Strickland <sstrickl@google.com>
43 lines
1.2 KiB
C++
43 lines
1.2 KiB
C++
// Copyright (c) 2018, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_HASH_H_
|
|
#define RUNTIME_VM_HASH_H_
|
|
|
|
#include "platform/globals.h"
|
|
|
|
namespace dart {
|
|
|
|
inline uint32_t CombineHashes(uint32_t hash, uint32_t other_hash) {
|
|
hash += other_hash;
|
|
hash += hash << 10;
|
|
hash ^= hash >> 6; // Logical shift, unsigned hash.
|
|
return hash;
|
|
}
|
|
|
|
inline uint32_t FinalizeHash(uint32_t hash, intptr_t hashbits = kBitsPerInt32) {
|
|
hash += hash << 3;
|
|
hash ^= hash >> 11; // Logical shift, unsigned hash.
|
|
hash += hash << 15;
|
|
// FinalizeHash gets called with values for hashbits that are bigger than 31
|
|
// (like kBitsPerWord - 1). Therefore we are careful to use a type
|
|
// (uintptr_t) big enough to avoid undefined behavior with the left shift.
|
|
hash &= (static_cast<uintptr_t>(1) << hashbits) - 1;
|
|
return (hash == 0) ? 1 : hash;
|
|
}
|
|
|
|
inline uint32_t HashBytes(const uint8_t* bytes, intptr_t size) {
|
|
uint32_t hash = size;
|
|
while (size > 0) {
|
|
hash = CombineHashes(hash, *bytes);
|
|
bytes++;
|
|
size--;
|
|
}
|
|
return hash;
|
|
}
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_HASH_H_
|