mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
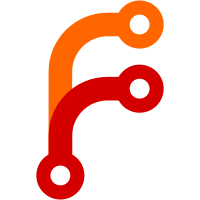
Small --optimization-counter-threshold makes tests very slow, especially on architectures where kernel service runs from kernel and not from app-jit snapshot. TEST=change in tests, *-ia32 bots Fixes https://github.com/dart-lang/sdk/issues/48627 Change-Id: I63e7e201ef9a0e4f645016c39a5be1819b61822d Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/263421 Commit-Queue: Alexander Markov <alexmarkov@google.com> Reviewed-by: Ryan Macnak <rmacnak@google.com>
42 lines
1,020 B
Dart
42 lines
1,020 B
Dart
// Copyright (c) 2014, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
//
|
|
// Dart Mint representations and type propagation issue.
|
|
// Testing Int32List and Uint32List loads.
|
|
//
|
|
// VMOptions=--optimization-counter-threshold=20 --no-use-osr --no-background-compilation
|
|
|
|
import 'dart:typed_data';
|
|
import "package:expect/expect.dart";
|
|
|
|
main() {
|
|
var a = new Uint32List(100);
|
|
a[2] = 3;
|
|
var res = sumIt1(a, 2);
|
|
Expect.equals(3 * 10, res);
|
|
res = sumIt1(a, 2);
|
|
Expect.equals(3 * 10, res);
|
|
var a1 = new Int32List(100);
|
|
a1[2] = 3;
|
|
res = sumIt2(a1, 2);
|
|
Expect.equals(3 * 10, res);
|
|
res = sumIt2(a1, 2);
|
|
Expect.equals(3 * 10, res);
|
|
}
|
|
|
|
sumIt1(Uint32List a, int n) {
|
|
var sum = 0;
|
|
for (int i = 0; i < 10; i++) {
|
|
sum += a[n];
|
|
}
|
|
return sum;
|
|
}
|
|
|
|
sumIt2(Int32List a, int n) {
|
|
var sum = 0;
|
|
for (int i = 0; i < 10; i++) {
|
|
sum += a[n];
|
|
}
|
|
return sum;
|
|
}
|