mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
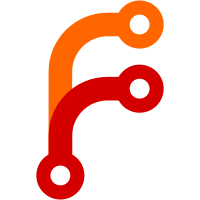
This allows building gen_snapshot with host=arm64, target=x64. TEST=ci Bug: https://github.com/flutter/flutter/issues/103386 Change-Id: I478cc0917462896de9b598455d2ed68401323b50 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/252962 Reviewed-by: Alexander Aprelev <aam@google.com> Reviewed-by: Siva Annamalai <asiva@google.com> Commit-Queue: Ryan Macnak <rmacnak@google.com>
76 lines
2 KiB
C++
76 lines
2 KiB
C++
// Copyright (c) 2022, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_SIMULATOR_X64_H_
|
|
#define RUNTIME_VM_SIMULATOR_X64_H_
|
|
|
|
#ifndef RUNTIME_VM_SIMULATOR_H_
|
|
#error Do not include simulator_x64.h directly; use simulator.h.
|
|
#endif
|
|
|
|
#include "vm/constants.h"
|
|
#include "vm/random.h"
|
|
|
|
namespace dart {
|
|
|
|
class Thread;
|
|
|
|
class Simulator {
|
|
public:
|
|
static const uword kSimulatorStackUnderflowSize = 64;
|
|
|
|
Simulator();
|
|
~Simulator();
|
|
|
|
static Simulator* Current();
|
|
|
|
int64_t Call(int64_t entry,
|
|
int64_t parameter0,
|
|
int64_t parameter1,
|
|
int64_t parameter2,
|
|
int64_t parameter3,
|
|
bool fp_return = false,
|
|
bool fp_args = false);
|
|
|
|
// Runtime and native call support.
|
|
enum CallKind {
|
|
kRuntimeCall,
|
|
kLeafRuntimeCall,
|
|
kLeafFloatRuntimeCall,
|
|
kNativeCallWrapper
|
|
};
|
|
static uword RedirectExternalReference(uword function,
|
|
CallKind call_kind,
|
|
int argument_count);
|
|
|
|
static uword FunctionForRedirect(uword redirect);
|
|
|
|
void JumpToFrame(uword pc, uword sp, uword fp, Thread* thread);
|
|
|
|
uint64_t get_register(Register rs) const { return 0; }
|
|
uint64_t get_pc() const { return 0; }
|
|
uint64_t get_sp() const { return 0; }
|
|
uint64_t get_fp() const { return 0; }
|
|
uint64_t get_lr() const { return 0; }
|
|
|
|
// High address.
|
|
uword stack_base() const { return 0; }
|
|
// Limit for StackOverflowError.
|
|
uword overflow_stack_limit() const { return 0; }
|
|
// Low address.
|
|
uword stack_limit() const { return 0; }
|
|
|
|
// Accessor to the instruction counter.
|
|
uint64_t get_icount() const { return 0; }
|
|
|
|
// Call on program start.
|
|
static void Init();
|
|
|
|
private:
|
|
DISALLOW_COPY_AND_ASSIGN(Simulator);
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_SIMULATOR_X64_H_
|