mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
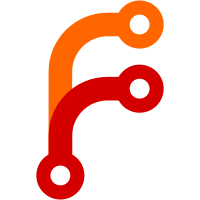
TEST=issue_4636_test.dart Bug: 46436,47402 Change-Id: I11579428f1d3c791ed64b7d5e6f75f41aef8c6c8 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/215981 Commit-Queue: Brian Quinlan <bquinlan@google.com> Reviewed-by: Alexander Aprelev <aam@google.com>
114 lines
3.1 KiB
C++
114 lines
3.1 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#include "platform/globals.h"
|
|
#if defined(DART_HOST_OS_ANDROID)
|
|
|
|
#include <errno.h> // NOLINT
|
|
#include <netdb.h> // NOLINT
|
|
#include <sys/time.h> // NOLINT
|
|
#include <time.h> // NOLINT
|
|
|
|
#include "bin/utils.h"
|
|
#include "platform/assert.h"
|
|
#include "platform/utils.h"
|
|
|
|
namespace dart {
|
|
namespace bin {
|
|
|
|
OSError::OSError() : sub_system_(kSystem), code_(0), message_(NULL) {
|
|
Reload();
|
|
}
|
|
|
|
void OSError::Reload() {
|
|
SetCodeAndMessage(kSystem, errno);
|
|
}
|
|
|
|
void OSError::SetCodeAndMessage(SubSystem sub_system, int code) {
|
|
set_sub_system(sub_system);
|
|
set_code(code);
|
|
if (sub_system == kSystem) {
|
|
const int kBufferSize = 1024;
|
|
char error_message[kBufferSize];
|
|
Utils::StrError(code, error_message, kBufferSize);
|
|
SetMessage(error_message);
|
|
} else if (sub_system == kGetAddressInfo) {
|
|
SetMessage(gai_strerror(code));
|
|
} else {
|
|
UNREACHABLE();
|
|
}
|
|
}
|
|
|
|
const char* StringUtils::ConsoleStringToUtf8(const char* str,
|
|
intptr_t len,
|
|
intptr_t* result_len) {
|
|
return NULL;
|
|
}
|
|
|
|
const char* StringUtils::Utf8ToConsoleString(const char* utf8,
|
|
intptr_t len,
|
|
intptr_t* result_len) {
|
|
return NULL;
|
|
}
|
|
|
|
char* StringUtils::ConsoleStringToUtf8(char* str,
|
|
intptr_t len,
|
|
intptr_t* result_len) {
|
|
return NULL;
|
|
}
|
|
|
|
char* StringUtils::Utf8ToConsoleString(char* utf8,
|
|
intptr_t len,
|
|
intptr_t* result_len) {
|
|
return NULL;
|
|
}
|
|
|
|
bool ShellUtils::GetUtf8Argv(int argc, char** argv) {
|
|
return false;
|
|
}
|
|
|
|
void TimerUtils::InitOnce() {}
|
|
|
|
int64_t TimerUtils::GetCurrentMonotonicMillis() {
|
|
return GetCurrentMonotonicMicros() / 1000;
|
|
}
|
|
|
|
int64_t TimerUtils::GetCurrentMonotonicMicros() {
|
|
struct timespec ts;
|
|
if (clock_gettime(CLOCK_MONOTONIC, &ts) != 0) {
|
|
UNREACHABLE();
|
|
return 0;
|
|
}
|
|
// Convert to microseconds.
|
|
int64_t result = ts.tv_sec;
|
|
result *= kMicrosecondsPerSecond;
|
|
result += (ts.tv_nsec / kNanosecondsPerMicrosecond);
|
|
return result;
|
|
}
|
|
|
|
void TimerUtils::Sleep(int64_t millis) {
|
|
struct timespec req; // requested.
|
|
struct timespec rem; // remainder.
|
|
int64_t micros = millis * kMicrosecondsPerMillisecond;
|
|
int64_t seconds = micros / kMicrosecondsPerSecond;
|
|
micros = micros - seconds * kMicrosecondsPerSecond;
|
|
int64_t nanos = micros * kNanosecondsPerMicrosecond;
|
|
req.tv_sec = seconds;
|
|
req.tv_nsec = nanos;
|
|
while (true) {
|
|
int r = nanosleep(&req, &rem);
|
|
if (r == 0) {
|
|
break;
|
|
}
|
|
// We should only ever see an interrupt error.
|
|
ASSERT(errno == EINTR);
|
|
// Copy remainder into requested and repeat.
|
|
req = rem;
|
|
}
|
|
}
|
|
|
|
} // namespace bin
|
|
} // namespace dart
|
|
|
|
#endif // defined(DART_HOST_OS_ANDROID)
|