mirror of
https://github.com/dart-lang/sdk
synced 2024-09-15 22:51:29 +00:00
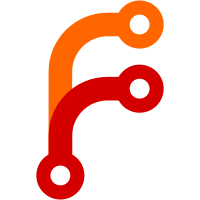
Changes: Doing this always in precompiled mode meant increased data segment sizes when CodeSourceMaps are stored, since encoded line/column information is larger in the LEB-like encoding used. Now we only store column information when we produce non-symbolic stacks, since the increased space needed to store the columns is instead in DWARF sections and can be stripped or elided. Original description: Previously, we passed line number information to the stack trace printer and to DWARF by changing the non-special positions in the CodeSourceMap to line numbers in precompiled mode. However, doing this lost column information. We get the column information back in the majority of cases by encoding the line number and column information when neither is too large to pack together into 30 bits. (Here, 20 bits for line and 10 bits for column.) Otherwise, we just store the line information as before, though due to using a bit to encode whether column info exists, it's reduced to 30 bits. If the line info is too big for that, we just return kNoSourcePos. Cq-Include-Trybots: luci.dart.try:vm-kernel-precomp-nnbd-linux-release-x64-try,vm-kernel-precomp-linux-release-x64-try,vm-kernel-precomp-linux-product-x64-try,vm-kernel-precomp-linux-debug-x64-try,vm-kernel-precomp-linux-release-simarm_x64-try Change-Id: Ia8baee71468da6100a170fa305d03059ffd17f78 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/151822 Commit-Queue: Tess Strickland <sstrickl@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com>
77 lines
2 KiB
Dart
77 lines
2 KiB
Dart
// Copyright (c) 2017, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
/// VMOptions=--dwarf-stack-traces --save-debugging-info=dwarf_obfuscate.so --obfuscate
|
|
|
|
import 'dart:io';
|
|
|
|
import 'package:native_stack_traces/native_stack_traces.dart';
|
|
import 'package:path/path.dart' as path;
|
|
|
|
import 'dwarf_stack_trace_test.dart' as base;
|
|
|
|
@pragma("vm:prefer-inline")
|
|
bar() {
|
|
// Keep the 'throw' and its argument on separate lines.
|
|
throw // force linebreak with dartfmt
|
|
"Hello, Dwarf!";
|
|
}
|
|
|
|
@pragma("vm:never-inline")
|
|
foo() {
|
|
bar();
|
|
}
|
|
|
|
Future<void> main() async {
|
|
String rawStack = "";
|
|
try {
|
|
foo();
|
|
} catch (e, st) {
|
|
rawStack = st.toString();
|
|
}
|
|
|
|
if (path.basenameWithoutExtension(Platform.executable) !=
|
|
"dart_precompiled_runtime") {
|
|
return; // Not running from an AOT compiled snapshot.
|
|
}
|
|
|
|
if (Platform.isAndroid) {
|
|
return; // Generated dwarf.so not available on the test device.
|
|
}
|
|
|
|
final dwarf = Dwarf.fromFile("dwarf_obfuscate.so");
|
|
|
|
await base.checkStackTrace(rawStack, dwarf, expectedCallsInfo);
|
|
}
|
|
|
|
final expectedCallsInfo = <List<DartCallInfo>>[
|
|
// The first frame should correspond to the throw in bar, which was inlined
|
|
// into foo (so we'll get information for two calls for that PC address).
|
|
[
|
|
DartCallInfo(
|
|
function: "bar",
|
|
filename: "dwarf_stack_trace_obfuscate_test.dart",
|
|
line: 17,
|
|
column: 3,
|
|
inlined: true),
|
|
DartCallInfo(
|
|
function: "foo",
|
|
filename: "dwarf_stack_trace_obfuscate_test.dart",
|
|
line: 23,
|
|
column: 3,
|
|
inlined: false)
|
|
],
|
|
// The second frame corresponds to call to foo in main.
|
|
[
|
|
DartCallInfo(
|
|
function: "main",
|
|
filename: "dwarf_stack_trace_obfuscate_test.dart",
|
|
line: 29,
|
|
column: 5,
|
|
inlined: false)
|
|
],
|
|
// Don't assume anything about any of the frames below the call to foo
|
|
// in main, as this makes the test too brittle.
|
|
];
|