mirror of
https://github.com/dart-lang/sdk
synced 2024-09-15 23:39:48 +00:00
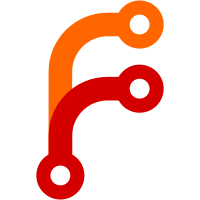
This CL introduces dart_api_dl.h which exposes a subset of dart_api.h and dart_native_api.h for dynamic linking at runtime through the FFI. Dynamic linking is done through including dart_api_dl.cc in a shared library and passing NativeApi.initializeApiDLData to the init function. This CL also includes Native API versioning to deal with possible version skew between native api version against which native libraries are compiled and the version in the DartVM the code is run on. The subset of symbols in the CL includes handle related symbols, error related symbols, handle scope symbols, and native port sumbols. Design: http://go/dart-ffi-expose-dart-api Closes: https://github.com/dart-lang/sdk/issues/40607 Closes: https://github.com/dart-lang/sdk/issues/36858 Closes: https://github.com/dart-lang/sdk/issues/41319 Closes: https://github.com/flutter/flutter/issues/46887 Closes: https://github.com/flutter/flutter/issues/47061 Misc: Closes: https://github.com/dart-lang/sdk/issues/42260 Change-Id: I9e557808dbc99b341f23964cbddbb05f26d7a6c5 Cq-Include-Trybots: luci.dart.try:vm-ffi-android-debug-arm-try,vm-ffi-android-debug-arm64-try,app-kernel-linux-debug-x64-try,vm-kernel-linux-debug-ia32-try,vm-kernel-win-debug-x64-try,vm-kernel-win-debug-ia32-try,vm-kernel-precomp-linux-debug-x64-try,vm-dartkb-linux-release-x64-abi-try,vm-kernel-precomp-android-release-arm64-try,vm-kernel-asan-linux-release-x64-try,vm-kernel-msan-linux-release-x64-try,vm-kernel-precomp-msan-linux-release-x64-try,vm-kernel-linux-release-simarm-try,vm-kernel-linux-release-simarm64-try,vm-kernel-precomp-android-release-arm_x64-try,vm-kernel-precomp-obfuscate-linux-release-x64-try,dart-sdk-linux-try,analyzer-analysis-server-linux-try,analyzer-linux-release-try,front-end-linux-release-x64-try,vm-kernel-precomp-win-release-x64-try,vm-kernel-mac-debug-x64-try,vm-precomp-ffi-qemu-linux-release-arm-try,vm-kernel-nnbd-linux-debug-x64-try,analyzer-nnbd-linux-release-try,front-end-nnbd-linux-release-x64-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/145592 Commit-Queue: Daco Harkes <dacoharkes@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com> Reviewed-by: Alexander Markov <alexmarkov@google.com>
126 lines
4.5 KiB
C
126 lines
4.5 KiB
C
/*
|
|
* Copyright (c) 2020, the Dart project authors. Please see the AUTHORS file
|
|
* for details. All rights reserved. Use of this source code is governed by a
|
|
* BSD-style license that can be found in the LICENSE file.
|
|
*/
|
|
|
|
#ifndef RUNTIME_INCLUDE_DART_API_DL_H_
|
|
#define RUNTIME_INCLUDE_DART_API_DL_H_
|
|
|
|
#include "include/dart_api.h"
|
|
#include "include/dart_native_api.h"
|
|
|
|
/** \mainpage Dynamically Linked Dart API
|
|
*
|
|
* This exposes a subset of symbols from dart_api.h and dart_native_api.h
|
|
* available in every Dart embedder through dynamic linking.
|
|
*
|
|
* All symbols are postfixed with _DL to indicate that they are dynamically
|
|
* linked and to prevent conflicts with the original symbol.
|
|
*
|
|
* Link `dart_api_dl.cc` file into your library and invoke
|
|
* `Dart_InitializeApiDL` with `NativeApi.initializeApiDLData`.
|
|
*/
|
|
|
|
intptr_t Dart_InitializeApiDL(void* data);
|
|
|
|
// IMPORTANT! Never update these signatures without properly updating
|
|
// DART_API_DL_MAJOR_VERSION and DART_API_DL_MINOR_VERSION.
|
|
//
|
|
// Verbatim copy of `dart_native_api.h` and `dart_api.h` symbols to trigger
|
|
// compile-time errors if the sybols in those files are updated without
|
|
// updating these.
|
|
//
|
|
// Function signatures and typedefs are carbon copied. Structs are typechecked
|
|
// nominally in C/C++, so they are not copied, instead a comment is added to
|
|
// their definition.
|
|
typedef int64_t Dart_Port_DL;
|
|
|
|
typedef void (*Dart_NativeMessageHandler_DL)(Dart_Port_DL dest_port_id,
|
|
Dart_CObject* message);
|
|
|
|
DART_EXTERN_C bool (*Dart_PostCObject_DL)(Dart_Port_DL port_id,
|
|
Dart_CObject* message);
|
|
|
|
DART_EXTERN_C bool (*Dart_PostInteger_DL)(Dart_Port_DL port_id,
|
|
int64_t message);
|
|
|
|
DART_EXTERN_C Dart_Port_DL (*Dart_NewNativePort_DL)(
|
|
const char* name,
|
|
Dart_NativeMessageHandler_DL handler,
|
|
bool handle_concurrently);
|
|
|
|
DART_EXTERN_C bool (*Dart_CloseNativePort_DL)(Dart_Port_DL native_port_id);
|
|
|
|
DART_EXTERN_C bool (*Dart_IsError_DL)(Dart_Handle handle);
|
|
|
|
DART_EXTERN_C bool (*Dart_IsApiError_DL)(Dart_Handle handle);
|
|
|
|
DART_EXTERN_C bool (*Dart_IsUnhandledExceptionError_DL)(Dart_Handle handle);
|
|
|
|
DART_EXTERN_C bool (*Dart_IsCompilationError_DL)(Dart_Handle handle);
|
|
|
|
DART_EXTERN_C bool (*Dart_IsFatalError_DL)(Dart_Handle handle);
|
|
|
|
DART_EXTERN_C const char* (*Dart_GetError_DL)(Dart_Handle handle);
|
|
|
|
DART_EXTERN_C bool (*Dart_ErrorHasException_DL)(Dart_Handle handle);
|
|
|
|
DART_EXTERN_C Dart_Handle (*Dart_ErrorGetException_DL)(Dart_Handle handle);
|
|
|
|
DART_EXTERN_C Dart_Handle (*Dart_ErrorGetStackTrace_DL)(Dart_Handle handle);
|
|
|
|
DART_EXTERN_C Dart_Handle (*Dart_NewApiError_DL)(const char* error);
|
|
|
|
DART_EXTERN_C Dart_Handle (*Dart_NewCompilationError_DL)(const char* error);
|
|
|
|
DART_EXTERN_C Dart_Handle (*Dart_NewUnhandledExceptionError_DL)(
|
|
Dart_Handle exception);
|
|
|
|
DART_EXTERN_C void (*Dart_PropagateError_DL)(Dart_Handle handle);
|
|
|
|
DART_EXTERN_C Dart_Handle (*Dart_ToString_DL)(Dart_Handle object);
|
|
|
|
DART_EXTERN_C bool (*Dart_IdentityEquals_DL)(Dart_Handle obj1,
|
|
Dart_Handle obj2);
|
|
|
|
DART_EXTERN_C Dart_Handle (*Dart_HandleFromPersistent_DL)(
|
|
Dart_PersistentHandle object);
|
|
|
|
DART_EXTERN_C Dart_Handle (*Dart_HandleFromWeakPersistent_DL)(
|
|
Dart_WeakPersistentHandle object);
|
|
|
|
DART_EXTERN_C Dart_PersistentHandle (*Dart_NewPersistentHandle_DL)(
|
|
Dart_Handle object);
|
|
|
|
DART_EXTERN_C void (*Dart_SetPersistentHandle_DL)(Dart_PersistentHandle obj1,
|
|
Dart_Handle obj2);
|
|
|
|
DART_EXTERN_C void (*Dart_DeletePersistentHandle_DL)(
|
|
Dart_PersistentHandle object);
|
|
|
|
DART_EXTERN_C Dart_WeakPersistentHandle (*Dart_NewWeakPersistentHandle_DL)(
|
|
Dart_Handle object,
|
|
void* peer,
|
|
intptr_t external_allocation_size,
|
|
Dart_WeakPersistentHandleFinalizer callback);
|
|
|
|
DART_EXTERN_C void (*Dart_DeleteWeakPersistentHandle_DL)(
|
|
Dart_WeakPersistentHandle object);
|
|
|
|
DART_EXTERN_C bool (*Dart_Post_DL)(Dart_Port_DL port_id, Dart_Handle object);
|
|
|
|
DART_EXTERN_C Dart_Handle (*Dart_NewSendPort_DL)(Dart_Port_DL port_id);
|
|
|
|
DART_EXTERN_C Dart_Handle (*Dart_SendPortGetId_DL)(Dart_Handle port,
|
|
Dart_Port_DL* port_id);
|
|
|
|
DART_EXTERN_C void (*Dart_EnterScope_DL)();
|
|
|
|
DART_EXTERN_C void (*Dart_ExitScope_DL)();
|
|
// IMPORTANT! Never update these signatures without properly updating
|
|
// DART_API_DL_MAJOR_VERSION and DART_API_DL_MINOR_VERSION.
|
|
//
|
|
// End of verbatim copy.
|
|
|
|
#endif /* RUNTIME_INCLUDE_DART_API_DL_H_ */ /* NOLINT */ |