mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
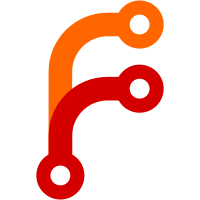
The problem with these changes was that closed ports still continue to receive messages and as a result we start using dead peer objects. Fixing that would require more intrusive changes into message handler implementation - so instead we are reverting the changes and restoring manual PORT -> PEER mapping. BUG= R=erikcorry@google.com Review-Url: https://codereview.chromium.org/2666063002 .
41 lines
1.2 KiB
C++
41 lines
1.2 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_NATIVE_MESSAGE_HANDLER_H_
|
|
#define RUNTIME_VM_NATIVE_MESSAGE_HANDLER_H_
|
|
|
|
#include "include/dart_api.h"
|
|
#include "include/dart_native_api.h"
|
|
#include "vm/message_handler.h"
|
|
|
|
namespace dart {
|
|
|
|
// A NativeMessageHandler accepts messages and dispatches them to
|
|
// native C handlers.
|
|
class NativeMessageHandler : public MessageHandler {
|
|
public:
|
|
NativeMessageHandler(const char* name, Dart_NativeMessageHandler func);
|
|
~NativeMessageHandler();
|
|
|
|
const char* name() const { return name_; }
|
|
Dart_NativeMessageHandler func() const { return func_; }
|
|
|
|
MessageStatus HandleMessage(Message* message);
|
|
|
|
#if defined(DEBUG)
|
|
// Check that it is safe to access this handler.
|
|
void CheckAccess();
|
|
#endif
|
|
|
|
// Delete this handlers when its last live port is closed.
|
|
virtual bool OwnedByPortMap() const { return true; }
|
|
|
|
private:
|
|
char* name_;
|
|
Dart_NativeMessageHandler func_;
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_NATIVE_MESSAGE_HANDLER_H_
|