mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 01:45:06 +00:00
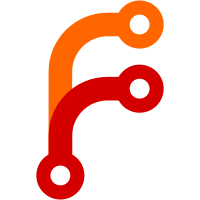
I was trying to debug an issue noticed that printing of LetNode is kind of useless. It didn't print the variables though they had references, which seems confusing, and it writes all the initializers and body nodes at the same nesting level which makes it impossible to see where one ends and the other begins. BUG= R=vegorov@google.com Review-Url: https://codereview.chromium.org/2946903002 .
59 lines
1.6 KiB
C++
59 lines
1.6 KiB
C++
// Copyright (c) 2011, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_AST_PRINTER_H_
|
|
#define RUNTIME_VM_AST_PRINTER_H_
|
|
|
|
#include "vm/ast.h"
|
|
#include "vm/growable_array.h"
|
|
|
|
namespace dart {
|
|
|
|
// Forward declaration.
|
|
class ParsedFunction;
|
|
class Log;
|
|
|
|
class AstPrinter : public AstNodeVisitor {
|
|
public:
|
|
explicit AstPrinter(bool log = true);
|
|
~AstPrinter();
|
|
|
|
void PrintNode(AstNode* node);
|
|
void PrintFunctionScope(const ParsedFunction& parsed_function);
|
|
void PrintFunctionNodes(const ParsedFunction& parsed_function);
|
|
|
|
#define DECLARE_VISITOR_FUNCTION(BaseName) \
|
|
virtual void Visit##BaseName##Node(BaseName##Node* node);
|
|
|
|
FOR_EACH_NODE(DECLARE_VISITOR_FUNCTION)
|
|
#undef DECLARE_VISITOR_FUNCTION
|
|
|
|
private:
|
|
static const int kScopeIndent = 2;
|
|
|
|
void IndentN(int count);
|
|
void PrintLocalScopeVariable(const LocalScope* scope,
|
|
LocalVariable* var,
|
|
int indent = 0);
|
|
void PrintLocalScope(const LocalScope* scope,
|
|
int variable_index,
|
|
int indent = 0);
|
|
|
|
void VisitGenericAstNode(AstNode* node);
|
|
void VisitGenericLocalNode(AstNode* node, const LocalVariable& local);
|
|
void VisitGenericFieldNode(AstNode* node, const Field& field);
|
|
|
|
void PrintLocalVariable(const LocalVariable* variable);
|
|
void PrintNewlineAndIndent();
|
|
|
|
intptr_t indent_;
|
|
Log* logger_;
|
|
|
|
DISALLOW_COPY_AND_ASSIGN(AstPrinter);
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_AST_PRINTER_H_
|