mirror of
https://github.com/dart-lang/sdk
synced 2024-09-18 21:51:18 +00:00
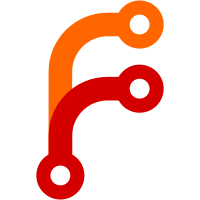
This changes analyzer to match the spec (and the VM behavior) with regard to which base class constructors are forwarded through mixin applications: only those constructors that take zero optional parameters are forwarded. In addition, the tests in tests/language/ are updated to match analyzer and the VM. There is one small difference between analyzer and the VM behavior: the spec says that if no constructors are forwarded at all, then the mixin application will automatically acquire an implicit default constructor. The VM behavior is to issue an error in this circumstance. After consulting with Gilad, it seems like the VM behavior is more consistent with the intent, so I've chosen to make analyzer and the tests match the VM behavior, in the hopes that the spec can be changed accordingly. Should we change our minds in the future and decide that we want to keep the spec as is, the tests and analyzer behavior can easily be changed. BUG=dartbug.com/19576 R=brianwilkerson@google.com Review URL: https://codereview.chromium.org//707073002 git-svn-id: https://dart.googlecode.com/svn/branches/bleeding_edge/dart@41645 260f80e4-7a28-3924-810f-c04153c831b5
37 lines
834 B
Dart
37 lines
834 B
Dart
// Copyright (c) 2014, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// Verify that a named mixin constructor forwards to the corresponding named
|
|
// base class constructor.
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
abstract class Mixin1 {
|
|
var mixin1Field = 1;
|
|
}
|
|
|
|
abstract class Mixin2 {
|
|
var mixin2Field = 2;
|
|
}
|
|
|
|
class A {
|
|
var superField;
|
|
A(foo) : superField = 0;
|
|
A.c1(foo) : superField = foo;
|
|
A.c2(foo) : superField = 0;
|
|
}
|
|
|
|
class B extends A with Mixin1, Mixin2 {
|
|
var field = 4;
|
|
B(unused) : super.c1(3);
|
|
}
|
|
|
|
main() {
|
|
var b = new B(null);
|
|
Expect.equals(1, b.mixin1Field);
|
|
Expect.equals(2, b.mixin2Field);
|
|
Expect.equals(3, b.superField);
|
|
Expect.equals(4, b.field);
|
|
}
|