mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 05:26:57 +00:00
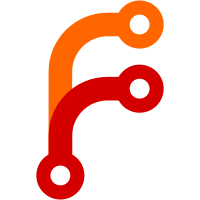
Followup to fb057ea4e0
Now that the web implementation of DateTime supports microseconds, the test special cases for not supporting microseconds can be removed.
Change-Id: I10991b25e42d643ae58850d7190621c9d11877b8
Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/367680
Reviewed-by: Lasse Nielsen <lrn@google.com>
Commit-Queue: Stephen Adams <sra@google.com>
40 lines
1.8 KiB
Dart
40 lines
1.8 KiB
Dart
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
check(DateTime expected, String str) {
|
|
DateTime actual = DateTime.parse(str);
|
|
Expect.equals(expected, actual); // Only checks if they are at the same time.
|
|
Expect.equals(expected.isUtc, actual.isUtc);
|
|
}
|
|
|
|
main() {
|
|
check(new DateTime(2012, 02, 27, 13, 27), "2012-02-27 13:27:00");
|
|
check(new DateTime.utc(2012, 02, 27, 13, 27, 0, 123, 456),
|
|
"2012-02-27 13:27:00.1234567891234z");
|
|
check(new DateTime.utc(2012, 02, 27, 13, 27, 0, 123, 456),
|
|
"2012-02-27 13:27:00,1234567891234z");
|
|
check(new DateTime(2012, 02, 27, 13, 27), "20120227 13:27:00");
|
|
check(new DateTime(2012, 02, 27, 13, 27), "20120227T132700");
|
|
check(new DateTime(2012, 02, 27), "20120227");
|
|
check(new DateTime(2012, 02, 27), "+20120227");
|
|
check(new DateTime.utc(2012, 02, 27, 14), "2012-02-27T14Z");
|
|
check(new DateTime.utc(-12345, 1, 1), "-123450101 00:00:00 Z");
|
|
check(new DateTime.utc(2012, 02, 27, 14), "2012-02-27T14+00");
|
|
check(new DateTime.utc(2012, 02, 27, 14), "2012-02-27T14+0000");
|
|
check(new DateTime.utc(2012, 02, 27, 14), "2012-02-27T14+00:00");
|
|
check(new DateTime.utc(2012, 02, 27, 14), "2012-02-27T14 +00:00");
|
|
|
|
check(new DateTime.utc(2015, 02, 14, 13, 0, 0, 0), "2015-02-15T00:00+11");
|
|
check(new DateTime.utc(2015, 02, 14, 13, 0, 0, 0), "2015-02-15T00:00:00+11");
|
|
check(
|
|
new DateTime.utc(2015, 02, 14, 13, 0, 0, 0), "2015-02-15T00:00:00+11:00");
|
|
|
|
check(new DateTime.utc(2015, 02, 15, 0, 0, 0, 500, 500),
|
|
"2015-02-15T00:00:00.500500Z");
|
|
check(new DateTime.utc(2015, 02, 15, 0, 0, 0, 511, 500),
|
|
"2015-02-15T00:00:00.511500Z");
|
|
}
|