mirror of
https://github.com/dart-lang/sdk
synced 2024-09-18 20:41:24 +00:00
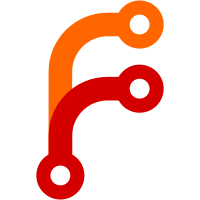
This allows the compiler to emit better errors. It also allows the compiler to detect when a class accidentally references its own supposedly disallowed constructors/operators (e.g., see dartutils.cc). Notably, this exposes a number of private member variables that are unused, but some that are used only in certain build configurations. It would arguably be better to only define the variables when they're needed, but that's deferred to a subsequent CL. Change-Id: I5d0e6697eebebc9321fae1ff49cc68caf557b903 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/97175 Commit-Queue: Siva Annamalai <asiva@google.com> Reviewed-by: Siva Annamalai <asiva@google.com>
277 lines
7 KiB
Plaintext
277 lines
7 KiB
Plaintext
# Copyright (c) 2014, the Dart project authors. Please see the AUTHORS file
|
|
# for details. All rights reserved. Use of this source code is governed by a
|
|
# BSD-style license that can be found in the LICENSE file.
|
|
|
|
import("../build/dart/dart_host_sdk_toolchain.gni")
|
|
import("configs.gni")
|
|
import("runtime_args.gni")
|
|
|
|
config("dart_public_config") {
|
|
include_dirs = [
|
|
".",
|
|
"include",
|
|
]
|
|
}
|
|
|
|
# Adds PRODUCT define if Flutter has specified "release" for dart_runtime_mode
|
|
config("dart_maybe_product_config") {
|
|
defines = []
|
|
|
|
if (dart_runtime_mode != "develop" && dart_runtime_mode != "profile" &&
|
|
dart_runtime_mode != "release") {
|
|
print("Invalid |dart_runtime_mode|")
|
|
assert(false)
|
|
}
|
|
|
|
if (dart_runtime_mode == "release") {
|
|
if (dart_debug) {
|
|
print("Debug and release mode are mutually exclusive.")
|
|
}
|
|
assert(!dart_debug)
|
|
defines += [ "PRODUCT" ]
|
|
}
|
|
}
|
|
|
|
# This is a config to use to build PRODUCT mode artifacts in a RELEASE build.
|
|
# If a DEBUG build has been specified it will be ignored.
|
|
config("dart_product_config") {
|
|
defines = []
|
|
if (!dart_debug) {
|
|
defines += [ "PRODUCT" ]
|
|
}
|
|
}
|
|
|
|
config("dart_precompiled_runtime_config") {
|
|
defines = []
|
|
defines += [
|
|
"DART_PRECOMPILED_RUNTIME",
|
|
"EXCLUDE_CFE_AND_KERNEL_PLATFORM",
|
|
]
|
|
}
|
|
|
|
# Controls DART_PRECOMPILER #define.
|
|
config("dart_precompiler_config") {
|
|
defines = []
|
|
defines += [ "DART_PRECOMPILER" ]
|
|
}
|
|
|
|
config("dart_nosnapshot_config") {
|
|
defines = []
|
|
defines += [ "DART_NO_SNAPSHOT" ]
|
|
}
|
|
|
|
config("dart_os_config") {
|
|
defines = []
|
|
|
|
# If dart_host_toolchain is the current toolchain, and it is different from
|
|
# host_toolchain, then we are building the SDK for the host, and should not
|
|
# hardcode these defines.
|
|
if (current_toolchain != dart_host_toolchain ||
|
|
host_toolchain == dart_host_toolchain) {
|
|
if (target_os == "android") {
|
|
defines += [ "TARGET_OS_ANDROID" ]
|
|
} else if (target_os == "fuchsia") {
|
|
defines += [ "TARGET_OS_FUCHSIA" ]
|
|
} else if (target_os == "ios") {
|
|
defines += [ "TARGET_OS_MACOS" ]
|
|
defines += [ "TARGET_OS_MACOS_IOS" ]
|
|
} else if (target_os == "linux") {
|
|
defines += [ "TARGET_OS_LINUX" ]
|
|
} else if (target_os == "mac") {
|
|
defines += [ "TARGET_OS_MACOS" ]
|
|
} else if (target_os == "win") {
|
|
defines += [ "TARGET_OS_WINDOWS" ]
|
|
} else {
|
|
print("Unknown target_os: $target_os")
|
|
assert(false)
|
|
}
|
|
}
|
|
}
|
|
|
|
# We need to build gen_snapshot targeting Fuchsia during a build of the SDK
|
|
# targeting Mac and Linux. This configuration is used to unconditionally target
|
|
# Fuchsia. It should not be combined with dart_os_config.
|
|
config("dart_os_fuchsia_config") {
|
|
defines = [ "TARGET_OS_FUCHSIA" ]
|
|
}
|
|
|
|
config("dart_arch_config") {
|
|
defines = []
|
|
|
|
# If dart_host_toolchain is the current toolchain, and it is different from
|
|
# host_toolchain, then we are building the SDK for the host, and should not
|
|
# hardcode these defines.
|
|
if (current_toolchain != dart_host_toolchain ||
|
|
host_toolchain == dart_host_toolchain) {
|
|
if (dart_target_arch == "arm") {
|
|
defines += [ "TARGET_ARCH_ARM" ]
|
|
} else if (dart_target_arch == "armv6") {
|
|
defines += [ "TARGET_ARCH_ARM" ]
|
|
defines += [ "TARGET_ARCH_ARM_6" ]
|
|
} else if (dart_target_arch == "armv5te") {
|
|
defines += [ "TARGET_ARCH_ARM" ]
|
|
defines += [ "TARGET_ARCH_ARM_5TE" ]
|
|
} else if (dart_target_arch == "arm64") {
|
|
defines += [ "TARGET_ARCH_ARM64" ]
|
|
} else if (dart_target_arch == "x64") {
|
|
defines += [ "TARGET_ARCH_X64" ]
|
|
} else if (dart_target_arch == "ia32" || dart_target_arch == "x86") {
|
|
defines += [ "TARGET_ARCH_IA32" ]
|
|
} else if (dart_target_arch == "dbc") {
|
|
defines += [ "TARGET_ARCH_DBC" ]
|
|
defines += [ "USING_SIMULATOR" ]
|
|
} else {
|
|
print("Invalid dart_target_arch: $dart_target_arch")
|
|
assert(false)
|
|
}
|
|
}
|
|
}
|
|
|
|
config("dart_config") {
|
|
defines = []
|
|
|
|
if (dart_debug) {
|
|
defines += [ "DEBUG" ]
|
|
} else {
|
|
defines += [ "NDEBUG" ]
|
|
}
|
|
|
|
include_dirs = [ "include" ]
|
|
if (dart_use_tcmalloc) {
|
|
defines += [ "DART_USE_TCMALLOC" ]
|
|
include_dirs += [ "../third_party/tcmalloc/gperftools/src" ]
|
|
}
|
|
|
|
if (is_fuchsia) {
|
|
include_dirs += [ "//zircon/system/ulib/zx/include" ]
|
|
}
|
|
|
|
if (!is_win) {
|
|
cflags = [
|
|
"-Werror",
|
|
"-Wall",
|
|
"-Wextra", # Also known as -W.
|
|
"-Wno-unused-parameter",
|
|
"-Wno-unused-private-field",
|
|
"-Wnon-virtual-dtor",
|
|
"-Wvla",
|
|
"-Wno-conversion-null",
|
|
"-Woverloaded-virtual",
|
|
"-Wno-comments", # Conflicts with clang-format.
|
|
"-g3",
|
|
"-ggdb3",
|
|
"-fno-rtti",
|
|
"-fno-exceptions",
|
|
"-Wimplicit-fallthrough",
|
|
]
|
|
|
|
ldflags = []
|
|
if (is_clang && dart_vm_code_coverage) {
|
|
cflags += [
|
|
"-O0",
|
|
"-fprofile-arcs",
|
|
"-ftest-coverage",
|
|
]
|
|
ldflags += [ "--coverage" ]
|
|
} else if (dart_debug) {
|
|
cflags += [ "-O${dart_debug_optimization_level}" ]
|
|
} else {
|
|
cflags += [ "-O3" ]
|
|
}
|
|
|
|
if (defined(is_asan) && is_asan) {
|
|
ldflags += [ "-fsanitize=address" ]
|
|
}
|
|
if (defined(is_msan) && is_msan) {
|
|
ldflags += [ "-fsanitize=memory" ]
|
|
}
|
|
if (defined(is_tsan) && is_tsan) {
|
|
ldflags += [ "-fsanitize=thread" ]
|
|
}
|
|
|
|
if (is_fuchsia) {
|
|
# safe-stack does not work with the interpreter.
|
|
cflags += [ "-fno-sanitize=safe-stack" ]
|
|
}
|
|
}
|
|
}
|
|
|
|
config("dart_shared_lib") {
|
|
if (dart_lib_export_symbols) {
|
|
defines = [ "DART_SHARED_LIB" ]
|
|
}
|
|
}
|
|
|
|
source_set("dart_api") {
|
|
public_configs = [ ":dart_public_config" ]
|
|
sources = [
|
|
"include/dart_api.h",
|
|
"include/dart_native_api.h",
|
|
"include/dart_tools_api.h",
|
|
]
|
|
}
|
|
|
|
library_for_all_configs("libdart") {
|
|
target_type = dart_component_kind
|
|
extra_deps = [
|
|
"third_party/double-conversion/src:libdouble_conversion",
|
|
":generate_version_cc_file",
|
|
]
|
|
if (is_fuchsia) {
|
|
extra_deps += [
|
|
"//zircon/public/lib/fbl",
|
|
"//zircon/public/lib/trace-engine",
|
|
]
|
|
}
|
|
configurable_deps = [
|
|
"platform:libdart_platform",
|
|
"vm:libdart_lib",
|
|
"vm:libdart_vm",
|
|
]
|
|
extra_configs = [ ":dart_shared_lib" ]
|
|
include_dirs = [ "." ]
|
|
public_configs = [ ":dart_public_config" ]
|
|
sources = [
|
|
"$target_gen_dir/version.cc",
|
|
"include/dart_api.h",
|
|
"include/dart_native_api.h",
|
|
"include/dart_tools_api.h",
|
|
"vm/dart_api_impl.cc",
|
|
"vm/native_api_impl.cc",
|
|
"vm/version.h",
|
|
]
|
|
}
|
|
|
|
action("generate_version_cc_file") {
|
|
inputs = [
|
|
"../tools/utils.py",
|
|
"../tools/VERSION",
|
|
"vm/version_in.cc",
|
|
]
|
|
if (dart_version_git_info) {
|
|
inputs += [ "../.git/logs/HEAD" ]
|
|
}
|
|
output = "$target_gen_dir/version.cc"
|
|
outputs = [
|
|
output,
|
|
]
|
|
|
|
script = "../tools/make_version.py"
|
|
args = [
|
|
"--quiet",
|
|
"--output",
|
|
rebase_path(output, root_build_dir),
|
|
"--input",
|
|
rebase_path("vm/version_in.cc", root_build_dir),
|
|
]
|
|
if (!dart_version_git_info) {
|
|
args += [ "--no_git_hash" ]
|
|
}
|
|
if (dart_custom_version_for_pub != "") {
|
|
args += [
|
|
"--custom_for_pub",
|
|
dart_custom_version_for_pub,
|
|
]
|
|
}
|
|
}
|