mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 16:41:07 +00:00
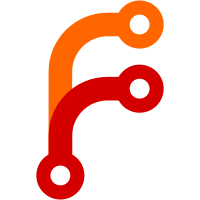
Change-Id: I46be49b2effec3e38a3dc44cd45cfe736f77fa78 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/182680 Commit-Queue: Sigmund Cherem <sigmund@google.com> Reviewed-by: Joshua Litt <joshualitt@google.com> Reviewed-by: Nicholas Shahan <nshahan@google.com> Reviewed-by: Stephen Adams <sra@google.com>
106 lines
2.2 KiB
Dart
106 lines
2.2 KiB
Dart
// Copyright (c) 2021, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'package:expect/expect.dart';
|
|
|
|
// Tests where late variable or field has a predictable value. An incorrect
|
|
// optimization might try to eliminate part of the check.
|
|
|
|
class C1 {
|
|
late String lastTag;
|
|
|
|
int zero(String tag) {
|
|
lastTag = tag;
|
|
return 0;
|
|
}
|
|
|
|
int one(String tag) {
|
|
lastTag = tag;
|
|
return 1;
|
|
}
|
|
|
|
int? returnNull(String tag) {
|
|
lastTag = tag;
|
|
return null;
|
|
}
|
|
|
|
late int? i1 = zero('C1.i1');
|
|
late int i2 = zero('C1.i2');
|
|
late int? i3 = returnNull('C1.i3');
|
|
late int i4 = one('C1.i4');
|
|
}
|
|
|
|
void test1() {
|
|
for (int i = 0; i < 10; i++) {
|
|
final c = C1();
|
|
|
|
Expect.equals(0, c.i1);
|
|
Expect.equals('C1.i1', c.lastTag);
|
|
Expect.equals(0, c.i2);
|
|
Expect.equals('C1.i2', c.lastTag);
|
|
Expect.equals(null, c.i3);
|
|
Expect.equals('C1.i3', c.lastTag);
|
|
|
|
Expect.equals(0, c.i1);
|
|
Expect.equals('C1.i3', c.lastTag);
|
|
Expect.equals(0, c.i2);
|
|
Expect.equals('C1.i3', c.lastTag);
|
|
Expect.equals(null, c.i3);
|
|
Expect.equals('C1.i3', c.lastTag);
|
|
|
|
Expect.equals(1, c.i4);
|
|
Expect.equals('C1.i4', c.lastTag);
|
|
}
|
|
}
|
|
|
|
class C2 {
|
|
late String lastTag;
|
|
|
|
int zero(String tag) {
|
|
lastTag = tag;
|
|
return 0;
|
|
}
|
|
|
|
int one(String tag) {
|
|
lastTag = tag;
|
|
return 1;
|
|
}
|
|
|
|
int? returnNull(String tag) {
|
|
lastTag = tag;
|
|
return null;
|
|
}
|
|
|
|
late final int? i1 = zero('C2.i1');
|
|
late final int i2 = zero('C2.i2');
|
|
late final int? i3 = returnNull('C2.i3');
|
|
late int i4 = one('C2.i4');
|
|
}
|
|
|
|
void test2() {
|
|
final c = C2();
|
|
|
|
Expect.equals(0, c.i1);
|
|
Expect.equals('C2.i1', c.lastTag);
|
|
Expect.equals(0, c.i2);
|
|
Expect.equals('C2.i2', c.lastTag);
|
|
Expect.equals(null, c.i3);
|
|
Expect.equals('C2.i3', c.lastTag);
|
|
|
|
Expect.equals(0, c.i1);
|
|
Expect.equals('C2.i3', c.lastTag);
|
|
Expect.equals(0, c.i2);
|
|
Expect.equals('C2.i3', c.lastTag);
|
|
Expect.equals(null, c.i3);
|
|
Expect.equals('C2.i3', c.lastTag);
|
|
|
|
Expect.equals(1, c.i4);
|
|
Expect.equals('C2.i4', c.lastTag);
|
|
}
|
|
|
|
main() {
|
|
test1();
|
|
test2();
|
|
}
|