mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 16:41:07 +00:00
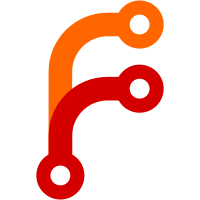
This CL adds the `Bool` `NativeType` with support for loading/storing from `Pointer<Bool>`, `Array<Bool>`, and `Struct` and `Union` fields, and support for passing booleans through FFI calls and callbacks. The assumption is that `bool` is always treated as `uint8_t` in the native ABIs. Including: (1) whether there can be garbage in the upper bytes in CPU registers, (2) stack alignment, and (3) alignment in compounds. The conversion from `bool` to `uint8_t` is implemented as follows: - bool to int: `value ? 1 : 0` - int to bool: `value != 0` The conversion is implemented in Dart in patch files for memory loads and stores (pointer, array, and struct fields) and kernel_to_il for FFI call and callback arguments and return value. TEST=runtime/vm/compiler/ffi/native_type_vm_test.cc TEST=tests/ffi/bool_test.dart TEST=tests/ffi/function_callbacks_structs_by_value_generated_test.dart TEST=tests/ffi/function_structs_by_value_generated_test.dart Closes: https://github.com/dart-lang/sdk/issues/36855 Change-Id: I75d100340ba41771abfb41c598ca92066a89370b Cq-Include-Trybots: luci.dart.try:vm-kernel-linux-debug-x64-try,vm-ffi-android-debug-arm-try,vm-kernel-mac-debug-x64-try,vm-kernel-nnbd-linux-debug-x64-try,vm-kernel-linux-debug-ia32-try,vm-kernel-precomp-linux-debug-x64-try,vm-kernel-reload-linux-debug-x64-try,vm-kernel-reload-rollback-linux-debug-x64-try,vm-kernel-win-debug-x64-try,vm-kernel-win-debug-ia32-try,vm-ffi-android-debug-arm64c-try,vm-kernel-mac-release-arm64-try,vm-precomp-ffi-qemu-linux-release-arm-try,vm-kernel-precomp-android-release-arm64c-try,vm-kernel-precomp-android-release-arm_x64-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/216900 Commit-Queue: Daco Harkes <dacoharkes@google.com> Reviewed-by: Ryan Macnak <rmacnak@google.com> Reviewed-by: Clement Skau <cskau@google.com>
92 lines
2 KiB
Dart
92 lines
2 KiB
Dart
// Copyright (c) 2021, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
//
|
|
// Dart test program for testing dart:ffi bools.
|
|
|
|
// @dart = 2.9
|
|
|
|
import 'dart:ffi';
|
|
|
|
import "package:expect/expect.dart";
|
|
import "package:ffi/ffi.dart";
|
|
|
|
import 'function_structs_by_value_generated_compounds.dart';
|
|
|
|
void main() {
|
|
testSizeOf();
|
|
testStoreLoad();
|
|
testStoreLoadIndexed();
|
|
testStruct();
|
|
testStruct2();
|
|
testInlineArray();
|
|
}
|
|
|
|
void testSizeOf() {
|
|
Expect.equals(1, sizeOf<Bool>());
|
|
}
|
|
|
|
void testStoreLoad() {
|
|
final p = calloc<Bool>();
|
|
p.value = true;
|
|
Expect.equals(true, p.value);
|
|
p.value = false;
|
|
Expect.equals(false, p.value);
|
|
calloc.free(p);
|
|
}
|
|
|
|
void testStoreLoadIndexed() {
|
|
final p = calloc<Bool>(2);
|
|
p[0] = true;
|
|
p[1] = false;
|
|
Expect.equals(true, p[0]);
|
|
Expect.equals(false, p[1]);
|
|
calloc.free(p);
|
|
}
|
|
|
|
void testStruct() {
|
|
final p = calloc<Struct1ByteBool>();
|
|
p.ref.a0 = true;
|
|
Expect.equals(true, p.ref.a0);
|
|
p.ref.a0 = false;
|
|
Expect.equals(false, p.ref.a0);
|
|
calloc.free(p);
|
|
}
|
|
|
|
void testStruct2() {
|
|
final p = calloc<Struct10BytesHomogeneousBool>();
|
|
p.ref.a0 = true;
|
|
p.ref.a1 = false;
|
|
p.ref.a2 = true;
|
|
p.ref.a3 = false;
|
|
p.ref.a4 = true;
|
|
p.ref.a5 = false;
|
|
p.ref.a6 = true;
|
|
p.ref.a7 = false;
|
|
p.ref.a8 = true;
|
|
p.ref.a9 = false;
|
|
Expect.equals(true, p.ref.a0);
|
|
Expect.equals(false, p.ref.a1);
|
|
Expect.equals(true, p.ref.a2);
|
|
Expect.equals(false, p.ref.a3);
|
|
Expect.equals(true, p.ref.a4);
|
|
Expect.equals(false, p.ref.a5);
|
|
Expect.equals(true, p.ref.a6);
|
|
Expect.equals(false, p.ref.a7);
|
|
Expect.equals(true, p.ref.a8);
|
|
Expect.equals(false, p.ref.a9);
|
|
calloc.free(p);
|
|
}
|
|
|
|
void testInlineArray() {
|
|
final p = calloc<Struct10BytesInlineArrayBool>();
|
|
final array = p.ref.a0;
|
|
for (int i = 0; i < 10; i++) {
|
|
array[i] = i % 2 == 0;
|
|
}
|
|
for (int i = 0; i < 10; i++) {
|
|
Expect.equals(i % 2 == 0, array[i]);
|
|
}
|
|
calloc.free(p);
|
|
}
|