mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 16:41:07 +00:00
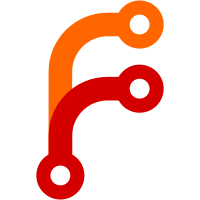
While deserializing AOT snapshot, Code objects which do not contain valuable information besides entry point and stack maps are discarded and not allocated on the heap (they are replaced with StubCode::UnknownDartCode()). PC -> Code/CompressedStackMaps lookup is implemented using a separate table (InstructionsTable). Flutter gallery in release-sizeopt mode: Heap size of snapshot objects: arm -26.89%, arm64 -27.68% Large Flutter application in release mode with --dwarf-stack-traces: Heap size of snapshot objects: -24.3%. Discarded Code objects: 72.5% of all Code objects. Issue: https://github.com/dart-lang/sdk/issues/44852. TEST=existing tests; "--dwarf_stack_traces --no-retain_function_objects --no-retain_code_objects" mode is enabled for a few tests. Change-Id: I5fe3e283630c8e8f4442319d5dcae38d174dd0d8 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/189560 Commit-Queue: Alexander Markov <alexmarkov@google.com> Reviewed-by: Ryan Macnak <rmacnak@google.com>
83 lines
2.3 KiB
Dart
83 lines
2.3 KiB
Dart
// Copyright (c) 2018, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// Testing Bigints with and without intrinsics.
|
|
// VMOptions=--intrinsify --no-enable-asserts
|
|
// VMOptions=--intrinsify --enable-asserts
|
|
// VMOptions=--no-intrinsify --enable-asserts
|
|
// VMOptions=--optimization-counter-threshold=5 --no-background-compilation
|
|
// VMOptions=--dwarf_stack_traces --no-retain_function_objects --no-retain_code_objects
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
import 'dart:math' show pow;
|
|
|
|
main() {
|
|
// Test integers.
|
|
testInt(0);
|
|
for (int i = 0; i < 63; i++) {
|
|
var n = pow(2, i) as int;
|
|
testInt(-n - 1);
|
|
testInt(-n);
|
|
testInt(-n + 1);
|
|
testInt(n - 1);
|
|
testInt(n);
|
|
testInt(n + 1);
|
|
}
|
|
testInt(-0x8000000000000000);
|
|
|
|
double dPow(num x, num exponent) => pow(x, exponent) as double;
|
|
|
|
// Test doubles.
|
|
testDouble(0.0);
|
|
testDouble(-0.0, 0.0);
|
|
for (double d in [
|
|
1.0,
|
|
2.0,
|
|
dPow(2.0, 30) - 1,
|
|
dPow(2.0, 30),
|
|
dPow(2.0, 31) - 1,
|
|
dPow(2.0, 31),
|
|
dPow(2.0, 31) + 1,
|
|
dPow(2.0, 32) - 1,
|
|
dPow(2.0, 32),
|
|
dPow(2.0, 32) + 1,
|
|
dPow(2.0, 52) - 1,
|
|
dPow(2.0, 52),
|
|
dPow(2.0, 52) + 1,
|
|
dPow(2.0, 53) - 1,
|
|
dPow(2.0, 53),
|
|
]) {
|
|
for (int p = 0; p < 1024; p++) {
|
|
var value = (d * pow(2.0, p)); // Valid integer value.
|
|
if (!value.isFinite) break;
|
|
testDouble(-value);
|
|
testDouble(value);
|
|
}
|
|
}
|
|
Expect.equals(BigInt.zero, new BigInt.from(0.5));
|
|
Expect.equals(BigInt.one, new BigInt.from(1.5));
|
|
|
|
Expect.throws(() => new BigInt.from(double.infinity));
|
|
Expect.throws(() => new BigInt.from(-double.infinity));
|
|
Expect.throws(() => new BigInt.from(double.nan));
|
|
}
|
|
|
|
testInt(int n) {
|
|
var bigint = new BigInt.from(n);
|
|
Expect.equals(n, bigint.toInt());
|
|
|
|
// If the integers are inexact (e.g. > 2^53 when represented by doubles as
|
|
// when compiled to JavaScript numbers) then the 'toString' might be rounded
|
|
// to the nearest equivalent 'nicer looking' number.
|
|
if (n == n + 1 || n == n - 1) return;
|
|
|
|
Expect.equals("$n", "$bigint");
|
|
}
|
|
|
|
testDouble(double input, [double? expectation]) {
|
|
var bigint = new BigInt.from(input);
|
|
Expect.equals(expectation ?? input, bigint.toDouble());
|
|
}
|